AI Dashboard is available on the Web, Apple, Google, and Microsoft, PRO version
QuickSort is an O(nlogn) efficient sorting algorithm, serving as systematic method for placing elements of an array in order. Quicksort is a comparison sort, meaning that it can sort items of any type for which a “less-than” relation (formally, a total order) is defined. In efficient implementations it is not a stable sort, meaning that the relative order of equal sort items is not preserved. Quicksort can operate in-place on an array, requiring small additional amounts of memory to perform the sorting. It is very similar to selection sort, except that it does not always choose worst-case partition.
Source: https://en.wikipedia.org/wiki/Quicksort
Below are 2 versions of the Quicksort Algorithm Implementation with Python. The first version is easy, but use more memory. The second version use the memory very efficiently.
I- QuickSort Algorithm Implementation with Python (Memory intensive version)

Output:
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes: 96DRHDRA9J7GTN6
Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE (Email us for more codes)
Active Anti-Aging Eye Gel, Reduces Dark Circles, Puffy Eyes, Crow's Feet and Fine Lines & Wrinkles, Packed with Hyaluronic Acid & Age Defying Botanicals
II- Quicksort Algorithm Implementation with Python (with Memory Optimisation)
III- QuickSort Algorithm Implementation with Python for Both Methods with duration captured
Output
III- Most Efficient Quicksort implementation in Python on array of integer represented as string. Example: array=[“1″,”237373737″,”3″,”1971771717171717″,”0”]
6 31415926535897932384626433832795 1 3 10 3 5
1 3 3 5 10 31415926535897932384626433832795 Just to clarify that lambda
part, in case someone else doesn't understand how exactly string comparison works:'2' > '1'
isTrue
, but'2' > '10'
is alsoTrue
, as well as'2' > '1000'
. That's why the strings are sorted by length first, becauselen('2') < len('10')
. IV- Build up a sorted array, one element at a time. Print the array after each iteration of the insertion sort, i.e., whenever the next element has been inserted at its correct position




Active Hydrating Toner, Anti-Aging Replenishing Advanced Face Moisturizer, with Vitamins A, C, E & Natural Botanicals to Promote Skin Balance & Collagen Production, 6.7 Fl Oz
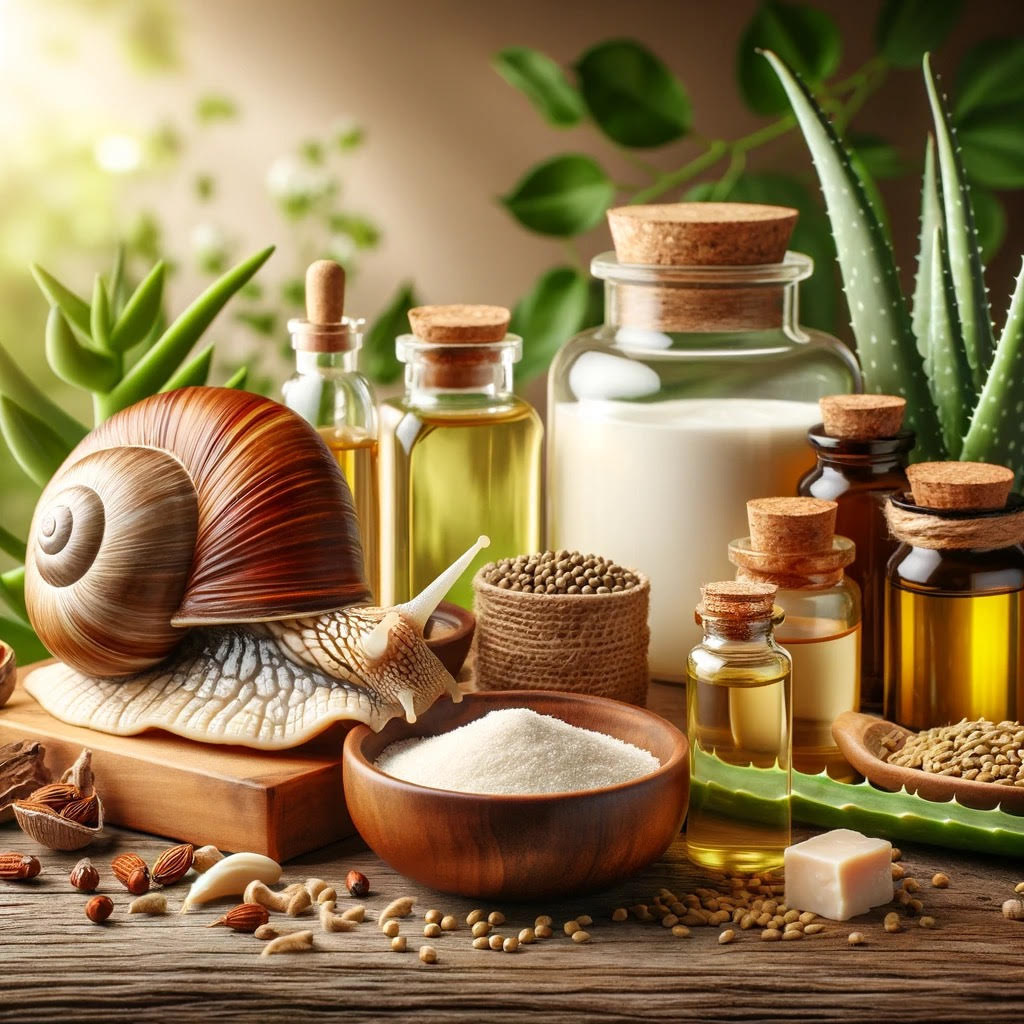

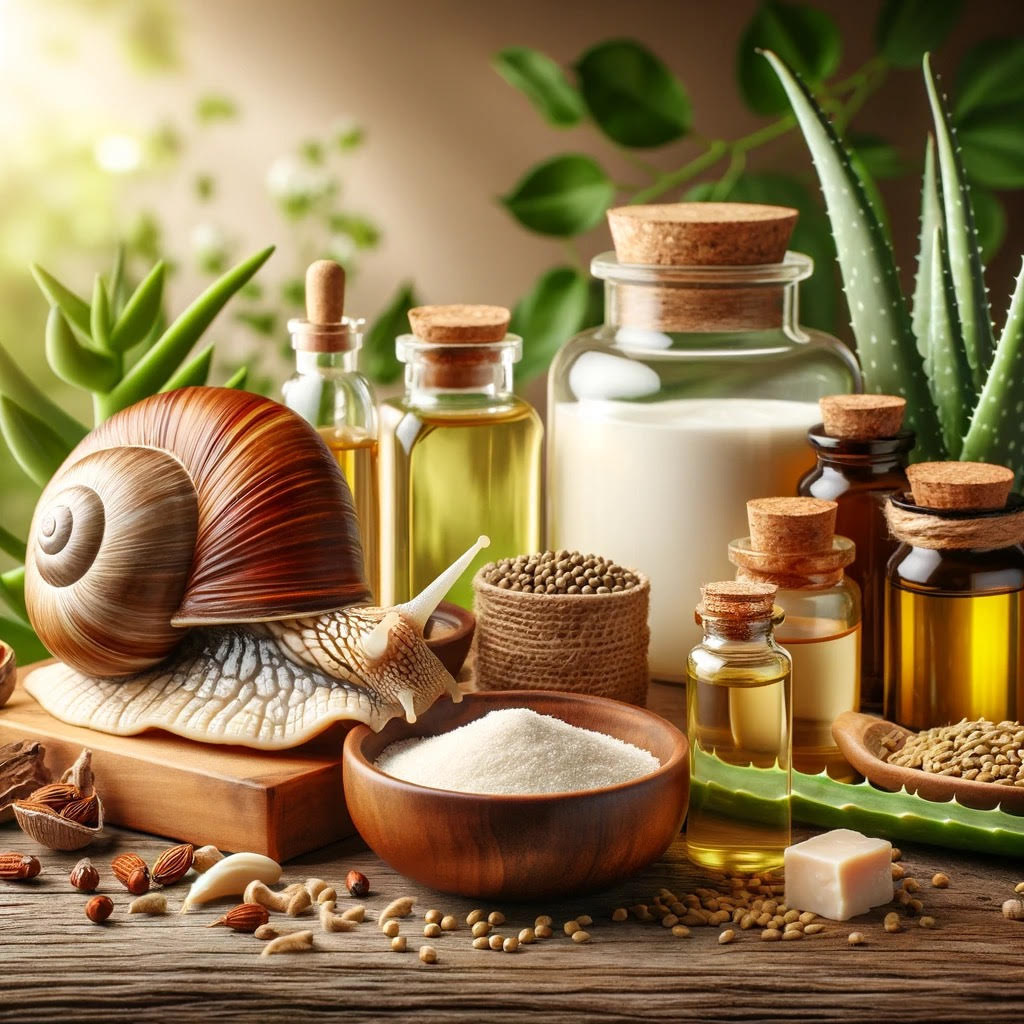

Age Defying 0.3% Retinol Serum, Anti-Aging Dark Spot Remover for Face, Fine Lines & Wrinkle Pore Minimizer, with Vitamin E & Natural Botanicals
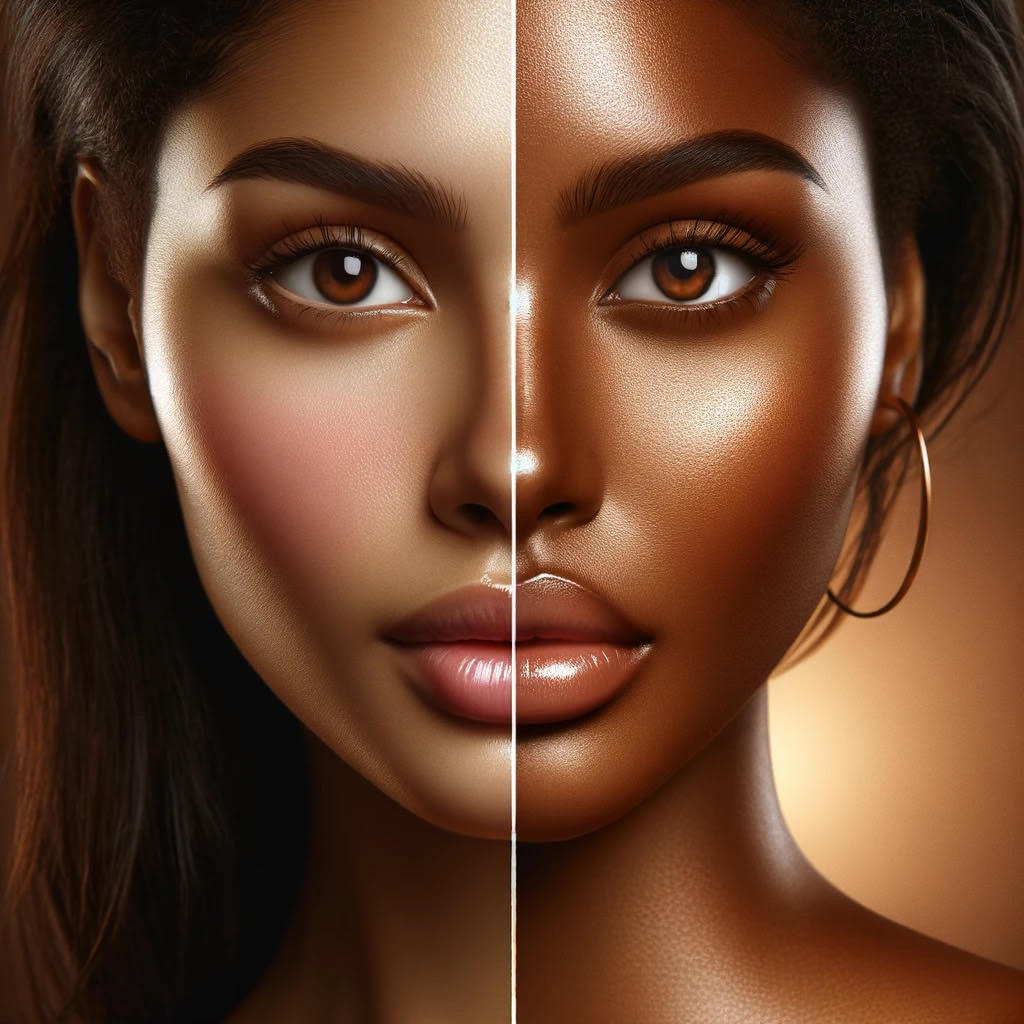

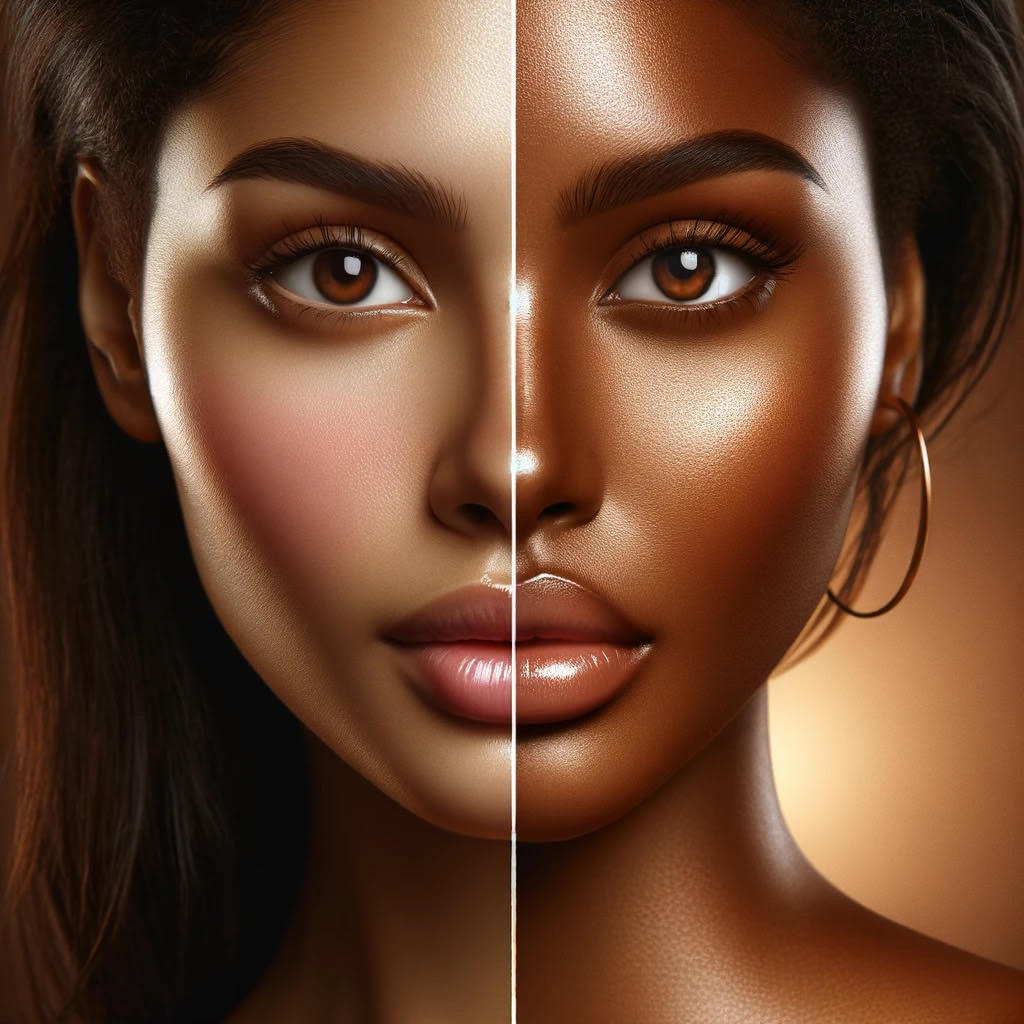

Firming Moisturizer, Advanced Hydrating Facial Replenishing Cream, with Hyaluronic Acid, Resveratrol & Natural Botanicals to Restore Skin's Strength, Radiance, and Resilience, 1.75 Oz


Skin Stem Cell Serum
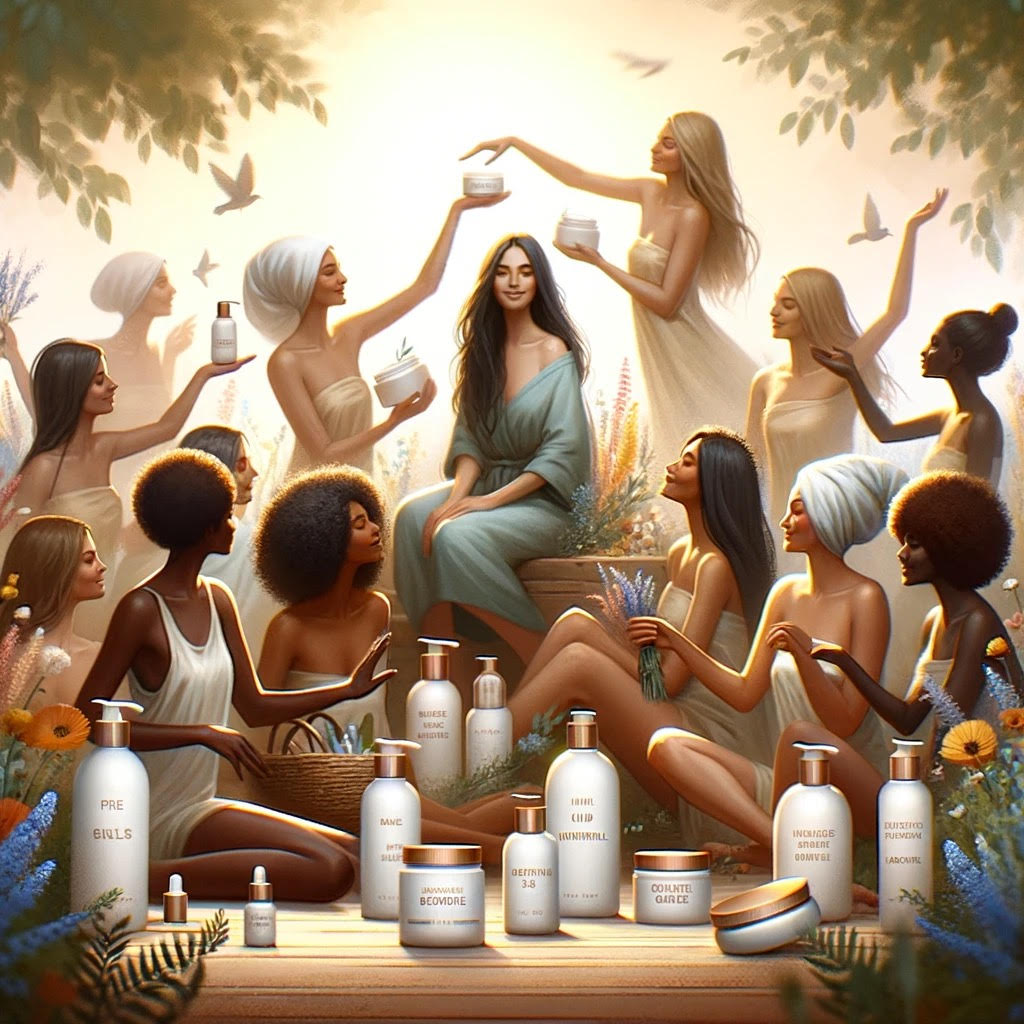

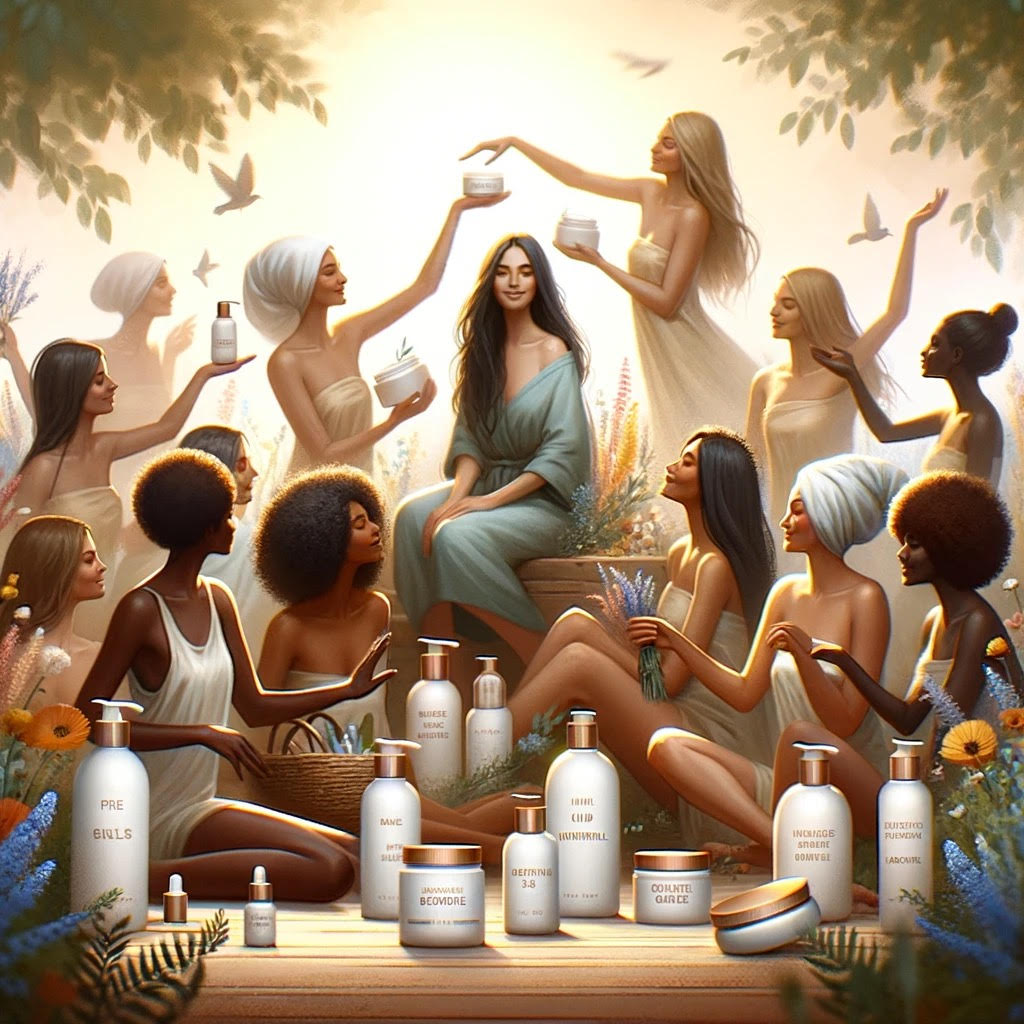

Smartphone 101 - Pick a smartphone for me - android or iOS - Apple iPhone or Samsung Galaxy or Huawei or Xaomi or Google Pixel
Can AI Really Predict Lottery Results? We Asked an Expert.
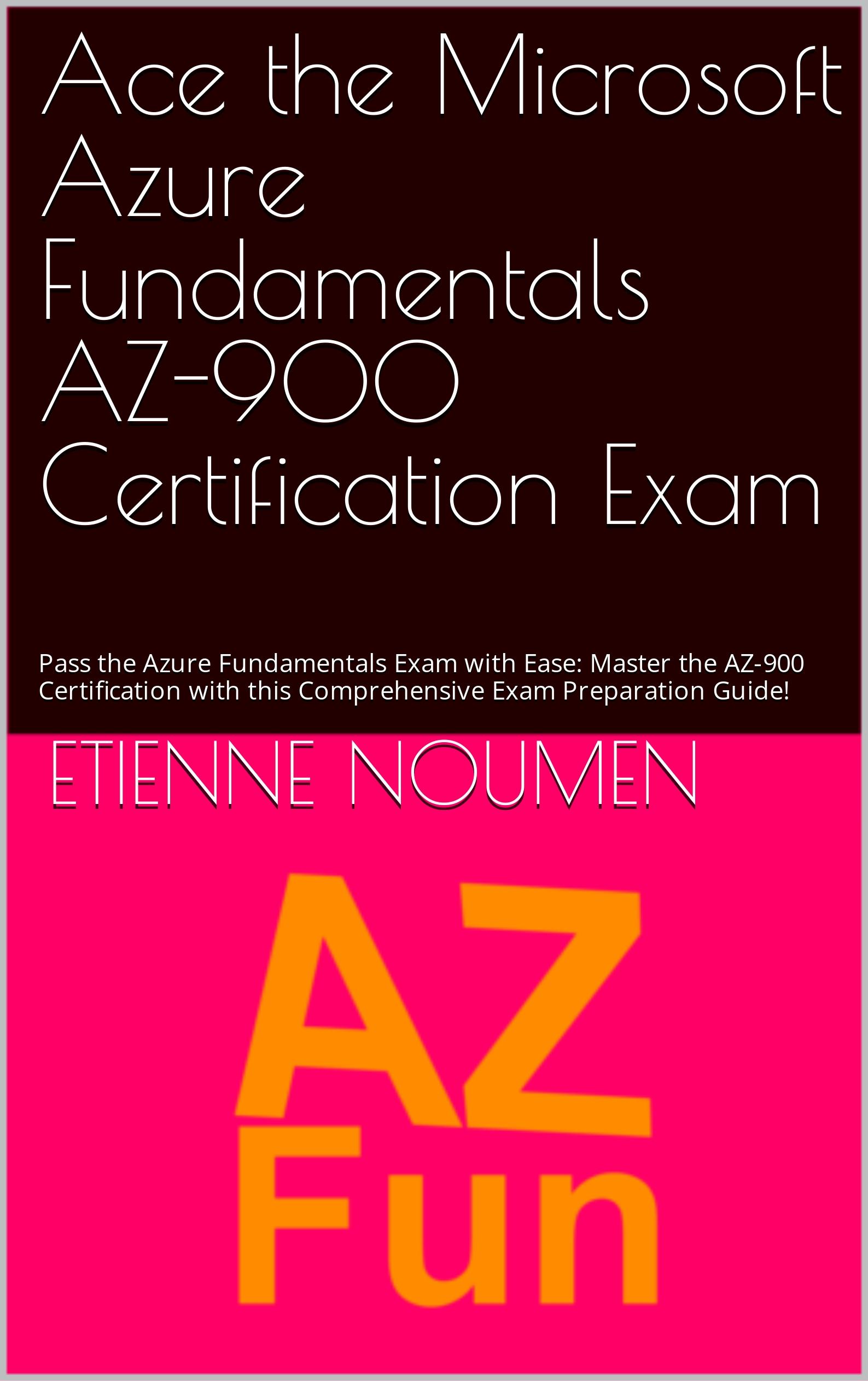
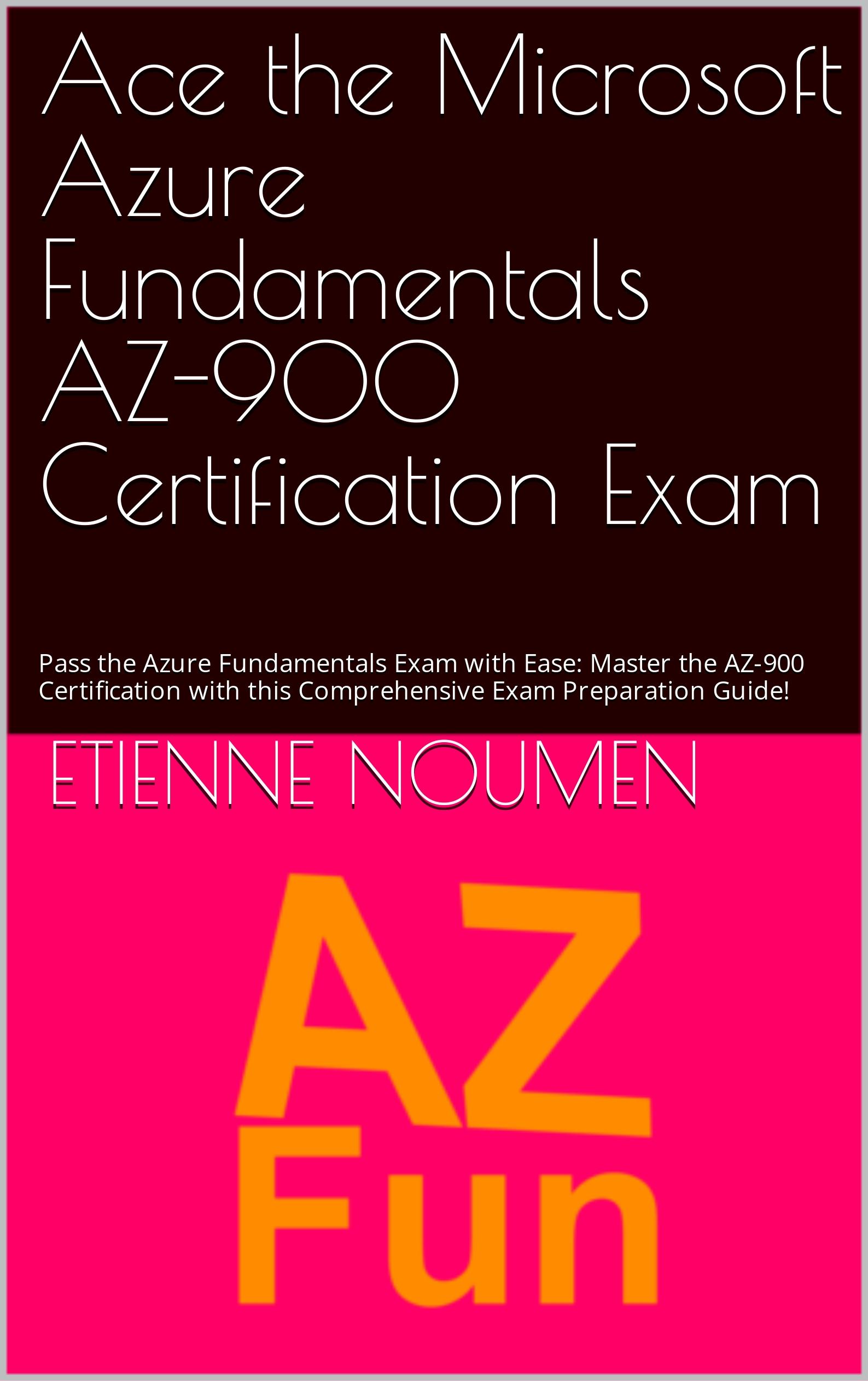


Djamgatech


Read Photos and PDFs Aloud for me iOS
Read Photos and PDFs Aloud for me android
Read Photos and PDFs Aloud For me Windows 10/11
Read Photos and PDFs Aloud For Amazon

Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE (Email us for more)
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes: 96DRHDRA9J7GTN6(Email us for more)

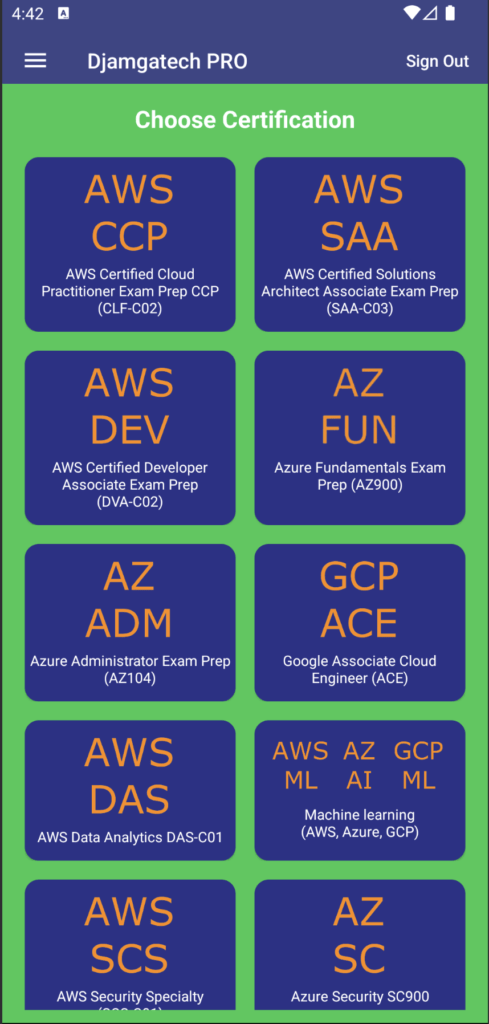
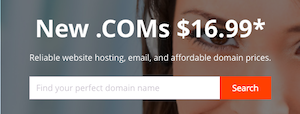
FREE 10000+ Quiz Trivia and and Brain Teasers for All Topics including Cloud Computing, General Knowledge, History, Television, Music, Art, Science, Movies, Films, US History, Soccer Football, World Cup, Data Science, Machine Learning, Geography, etc....
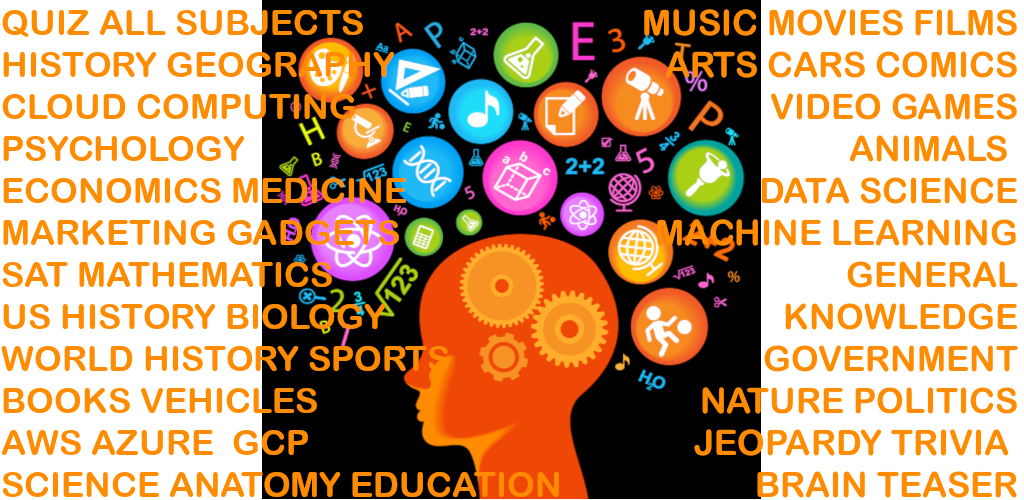

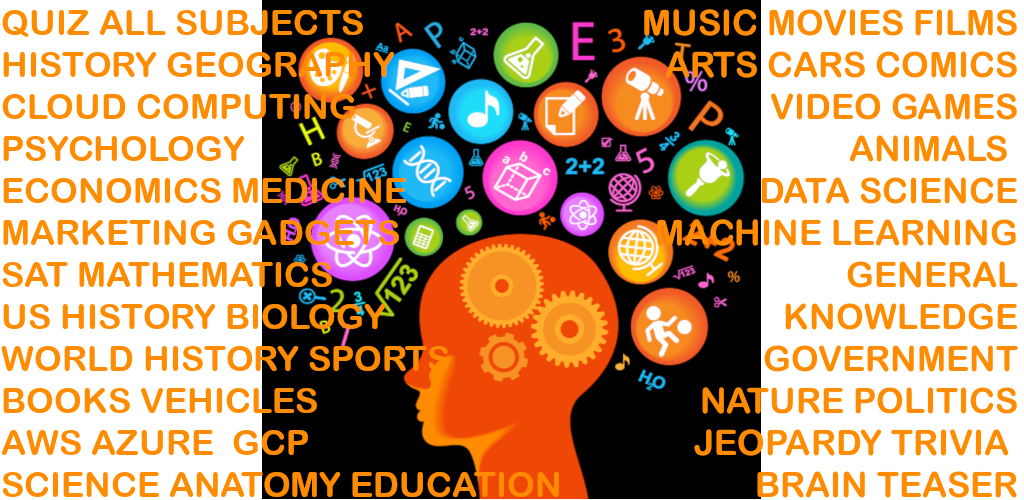
List of Freely available programming books - What is the single most influential book every Programmers should read
- Bjarne Stroustrup - The C++ Programming Language
- Brian W. Kernighan, Rob Pike - The Practice of Programming
- Donald Knuth - The Art of Computer Programming
- Ellen Ullman - Close to the Machine
- Ellis Horowitz - Fundamentals of Computer Algorithms
- Eric Raymond - The Art of Unix Programming
- Gerald M. Weinberg - The Psychology of Computer Programming
- James Gosling - The Java Programming Language
- Joel Spolsky - The Best Software Writing I
- Keith Curtis - After the Software Wars
- Richard M. Stallman - Free Software, Free Society
- Richard P. Gabriel - Patterns of Software
- Richard P. Gabriel - Innovation Happens Elsewhere
- Code Complete (2nd edition) by Steve McConnell
- The Pragmatic Programmer
- Structure and Interpretation of Computer Programs
- The C Programming Language by Kernighan and Ritchie
- Introduction to Algorithms by Cormen, Leiserson, Rivest & Stein
- Design Patterns by the Gang of Four
- Refactoring: Improving the Design of Existing Code
- The Mythical Man Month
- The Art of Computer Programming by Donald Knuth
- Compilers: Principles, Techniques and Tools by Alfred V. Aho, Ravi Sethi and Jeffrey D. Ullman
- Gödel, Escher, Bach by Douglas Hofstadter
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
- Effective C++
- More Effective C++
- CODE by Charles Petzold
- Programming Pearls by Jon Bentley
- Working Effectively with Legacy Code by Michael C. Feathers
- Peopleware by Demarco and Lister
- Coders at Work by Peter Seibel
- Surely You're Joking, Mr. Feynman!
- Effective Java 2nd edition
- Patterns of Enterprise Application Architecture by Martin Fowler
- The Little Schemer
- The Seasoned Schemer
- Why's (Poignant) Guide to Ruby
- The Inmates Are Running The Asylum: Why High Tech Products Drive Us Crazy and How to Restore the Sanity
- The Art of Unix Programming
- Test-Driven Development: By Example by Kent Beck
- Practices of an Agile Developer
- Don't Make Me Think
- Agile Software Development, Principles, Patterns, and Practices by Robert C. Martin
- Domain Driven Designs by Eric Evans
- The Design of Everyday Things by Donald Norman
- Modern C++ Design by Andrei Alexandrescu
- Best Software Writing I by Joel Spolsky
- The Practice of Programming by Kernighan and Pike
- Pragmatic Thinking and Learning: Refactor Your Wetware by Andy Hunt
- Software Estimation: Demystifying the Black Art by Steve McConnel
- The Passionate Programmer (My Job Went To India) by Chad Fowler
- Hackers: Heroes of the Computer Revolution
- Algorithms + Data Structures = Programs
- Writing Solid Code
- JavaScript - The Good Parts
- Getting Real by 37 Signals
- Foundations of Programming by Karl Seguin
- Computer Graphics: Principles and Practice in C (2nd Edition)
- Thinking in Java by Bruce Eckel
- The Elements of Computing Systems
- Refactoring to Patterns by Joshua Kerievsky
- Modern Operating Systems by Andrew S. Tanenbaum
- The Annotated Turing
- Things That Make Us Smart by Donald Norman
- The Timeless Way of Building by Christopher Alexander
- The Deadline: A Novel About Project Management by Tom DeMarco
- The C++ Programming Language (3rd edition) by Stroustrup
- Patterns of Enterprise Application Architecture
- Computer Systems - A Programmer's Perspective
- Agile Principles, Patterns, and Practices in C# by Robert C. Martin
- Growing Object-Oriented Software, Guided by Tests
- Framework Design Guidelines by Brad Abrams
- Object Thinking by Dr. David West
- Advanced Programming in the UNIX Environment by W. Richard Stevens
- Hackers and Painters: Big Ideas from the Computer Age
- The Soul of a New Machine by Tracy Kidder
- CLR via C# by Jeffrey Richter
- The Timeless Way of Building by Christopher Alexander
- Design Patterns in C# by Steve Metsker
- Alice in Wonderland by Lewis Carol
- Zen and the Art of Motorcycle Maintenance by Robert M. Pirsig
- About Face - The Essentials of Interaction Design
- Here Comes Everybody: The Power of Organizing Without Organizations by Clay Shirky
- The Tao of Programming
- Computational Beauty of Nature
- Writing Solid Code by Steve Maguire
- Philip and Alex's Guide to Web Publishing
- Object-Oriented Analysis and Design with Applications by Grady Booch
- Effective Java by Joshua Bloch
- Computability by N. J. Cutland
- Masterminds of Programming
- The Tao Te Ching
- The Productive Programmer
- The Art of Deception by Kevin Mitnick
- The Career Programmer: Guerilla Tactics for an Imperfect World by Christopher Duncan
- Paradigms of Artificial Intelligence Programming: Case studies in Common Lisp
- Masters of Doom
- Pragmatic Unit Testing in C# with NUnit by Andy Hunt and Dave Thomas with Matt Hargett
- How To Solve It by George Polya
- The Alchemist by Paulo Coelho
- Smalltalk-80: The Language and its Implementation
- Writing Secure Code (2nd Edition) by Michael Howard
- Introduction to Functional Programming by Philip Wadler and Richard Bird
- No Bugs! by David Thielen
- Rework by Jason Freid and DHH
- JUnit in Action
#BlackOwned #BlackEntrepreneurs #BlackBuniness #AWSCertified #AWSCloudPractitioner #AWSCertification #AWSCLFC02 #CloudComputing #AWSStudyGuide #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AWSBasics #AWSCertified #AWSMachineLearning #AWSCertification #AWSSpecialty #MachineLearning #AWSStudyGuide #CloudComputing #DataScience #AWSCertified #AWSSolutionsArchitect #AWSArchitectAssociate #AWSCertification #AWSStudyGuide #CloudComputing #AWSArchitecture #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AzureFundamentals #AZ900 #MicrosoftAzure #ITCertification #CertificationPrep #StudyMaterials #TechLearning #MicrosoftCertified #AzureCertification #TechBooks
Top 1000 Canada Quiz and trivia: CANADA CITIZENSHIP TEST- HISTORY - GEOGRAPHY - GOVERNMENT- CULTURE - PEOPLE - LANGUAGES - TRAVEL - WILDLIFE - HOCKEY - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION



Top 1000 Africa Quiz and trivia: HISTORY - GEOGRAPHY - WILDLIFE - CULTURE - PEOPLE - LANGUAGES - TRAVEL - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION
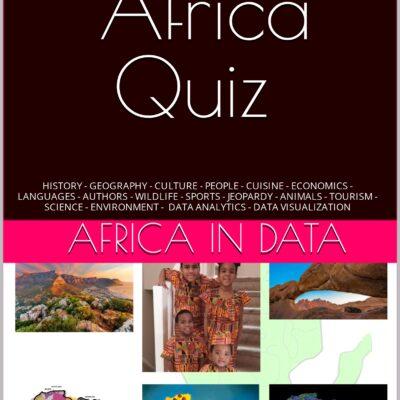

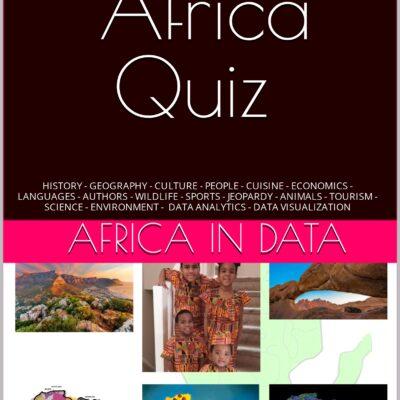
Exploring the Pros and Cons of Visiting All Provinces and Territories in Canada.



Exploring the Advantages and Disadvantages of Visiting All 50 States in the USA



Health Health, a science-based community to discuss health news and the coronavirus (COVID-19) pandemic
- Irregular bone marrow cells may increase heart disease riskby /u/Passervore on April 26, 2024 at 2:55 pm
submitted by /u/Passervore [link] [comments]
- ‘Real hope’ for cancer cure as personal mRNA vaccine for melanoma trialledby /u/Well_Socialized on April 26, 2024 at 2:53 pm
submitted by /u/Well_Socialized [link] [comments]
- 20% of grocery store milk has traces of bird flu, suggesting wider outbreak | The milk is still considered safe, but disease experts are alarmed by the prevalence.by /u/chrisdh79 on April 26, 2024 at 2:48 pm
submitted by /u/chrisdh79 [link] [comments]
- "Teachers and family dismissed my cry for help—it was almost too late"by /u/newsweek on April 26, 2024 at 12:41 pm
submitted by /u/newsweek [link] [comments]
- A new kind of gene-edited pig kidney was just transplanted into a personby /u/Sariel007 on April 26, 2024 at 12:32 pm
submitted by /u/Sariel007 [link] [comments]
Today I Learned (TIL) You learn something new every day; what did you learn today? Submit interesting and specific facts about something that you just found out here.
- TIL A group of horses were trained to communicate whether they wanted a jacket. All horses in the group successfully communicated that they did want a jacket when it was cold and did not want a jacket when it was hot.by /u/PunnyBanana on April 26, 2024 at 2:20 pm
submitted by /u/PunnyBanana [link] [comments]
- TIL when the artists arrived to record "We Are the World," Stevie Wonder told them that if the song wasn't finished in one take, he and Ray Charles would drive them home.by /u/RequirementSouth4482 on April 26, 2024 at 1:53 pm
submitted by /u/RequirementSouth4482 [link] [comments]
- TIL E.T was a 12-year-old disabled boy in a suitby /u/DiaBoloix on April 26, 2024 at 12:43 pm
submitted by /u/DiaBoloix [link] [comments]
- TIL that a politician gave a food review of kebab while speaking in parliament. Australian Senator Sam Dastyari gave a "10 out of 10" rating to the kebab snack pack sold at King Kebab House, and advised others to also enjoy "a great Australian tradition of meat in a box".by /u/TMWNN on April 26, 2024 at 11:47 am
submitted by /u/TMWNN [link] [comments]
- TIL the infamous "Jump the Shark" episode of Happy Days (Season 5, Episode 3) was created as a way to showcase Henry Winkler's real-life water skiing skills. The episode drew over 30 million viewers.by /u/ColeBelthazorTurner on April 26, 2024 at 11:36 am
submitted by /u/ColeBelthazorTurner [link] [comments]
Reddit Science This community is a place to share and discuss new scientific research. Read about the latest advances in astronomy, biology, medicine, physics, social science, and more. Find and submit new publications and popular science coverage of current research.
- Researchers have developed a nanomaterial that could be used to treat neurodegenerative diseases, such as Alzheimer's or Parkinson's. The new "protein-like polymer" has been shown to alter the interaction between two key brain proteins in cell cultures, releasing an important antioxidant on demand.by /u/alexbeadlesci on April 26, 2024 at 3:34 pm
submitted by /u/alexbeadlesci [link] [comments]
- EV drivers need to transition from the “monitor fuel gauge model” (driver refuels when fuel is running out) which represents how most people refuel a petrol or diesel car, to the “event-triggered model” (driver plugs in as soon as arriving home or work) which is optimum for EV use, finds new study.by /u/mvea on April 26, 2024 at 1:49 pm
submitted by /u/mvea [link] [comments]
- Recent research challenges the common belief that childhood trauma affects the experience of ayahuasca, a plant-based psychedelic. Surprisingly, the study finds no connection between prior childhood trauma and the intensity of challenges faced when under the influence of ayahuasca.by /u/mvea on April 26, 2024 at 1:29 pm
submitted by /u/mvea [link] [comments]
- ‘Uncharted territory’: Dual fusion breakthrough in generating denser and safer plasmaby /u/Cleancoolenergy on April 26, 2024 at 1:12 pm
submitted by /u/Cleancoolenergy [link] [comments]
- Researchers have found a fast, and inexpensive way to create geometric patterns in carbon nanotube films. The resulting films turned out to have superior properties for manufacturing components for 6G communication devices and flexible and transparent electronics — such as wearable health trackers.by /u/Skoltech_ on April 26, 2024 at 11:56 am
submitted by /u/Skoltech_ [link] [comments]
Reddit Sports Sports News and Highlights from the NFL, NBA, NHL, MLB, MLS, and leagues around the world.
- Falcons GM explains shocking selection of Michael Penix Jr. that left Kirk Cousins 'disappointed'by /u/Oldtimer_2 on April 26, 2024 at 1:45 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Joel Embiid scores 50 points to lead 76ers past Knicks 125-114 to cut deficit to 2-1by /u/Oldtimer_2 on April 26, 2024 at 1:13 pm
submitted by /u/Oldtimer_2 [link] [comments]
- 49ers excited to have Aiyuk, Deebo, Pearsall togetherby /u/Oldtimer_2 on April 26, 2024 at 1:12 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Nuggets breeze past Lakers, take 3-0 series leadby /u/Oldtimer_2 on April 26, 2024 at 1:10 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Brazil legend Marta to retire from international footballby /u/PrincessBananas85 on April 26, 2024 at 1:08 pm
submitted by /u/PrincessBananas85 [link] [comments]
Turn your dream into reality with Google Workspace: It’s free for the first 14 days.
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes:
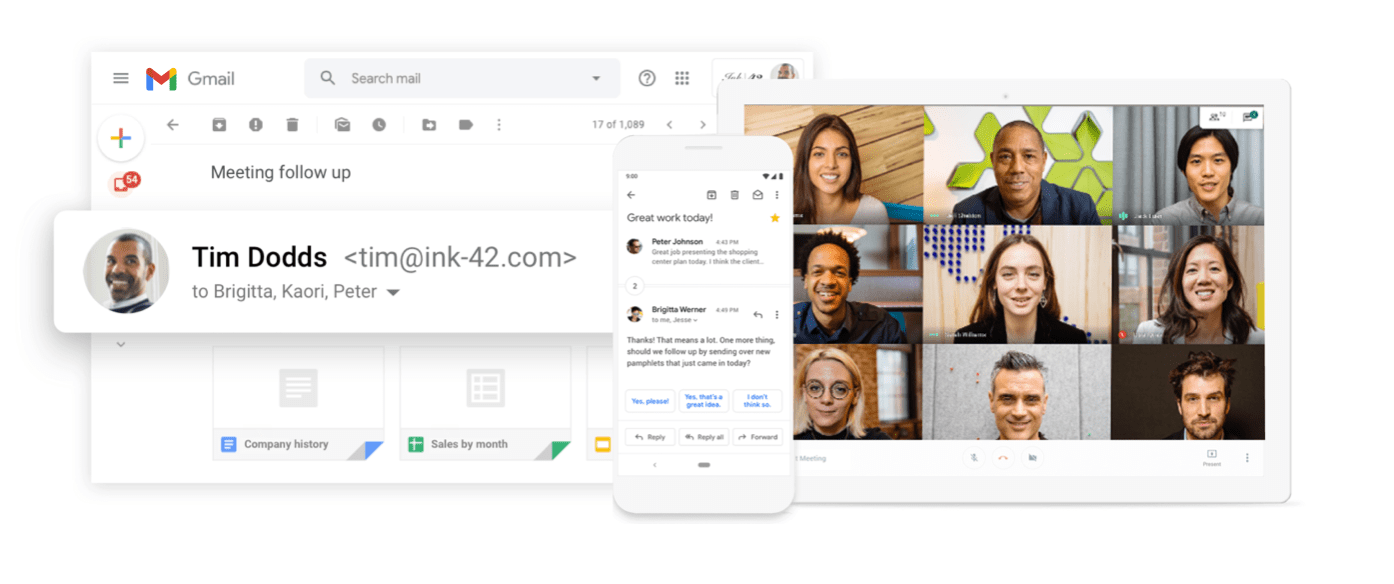

96DRHDRA9J7GTN6
63F733CLLY7R7MM
63F7D7CPD9XXUVT
63FLKQHWV3AEEE6
63JGLWWK36CP7WM
63KKR9EULQRR7VE
63KNY4N7VHCUA9R
63LDXXFYU6VXDG9
63MGNRCKXURAYWC
63NGNDVVXJP4N99
63P4G3ELRPADKQU
With Google Workspace, Get custom email @yourcompany, Work from anywhere; Easily scale up or down
Google gives you the tools you need to run your business like a pro. Set up custom email, share files securely online, video chat from any device, and more.
Google Workspace provides a platform, a common ground, for all our internal teams and operations to collaboratively support our primary business goal, which is to deliver quality information to our readers quickly.
Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE
C37HCAQRVR7JTFK
C3AE76E7WATCTL9
C3C3RGUF9VW6LXE
C3D9LD4L736CALC
C3EQXV674DQ6PXP
C3G9M3JEHXM3XC7
C3GGR3H4TRHUD7L
C3LVUVC3LHKUEQK
C3PVGM4CHHPMWLE
C3QHQ763LWGTW4C
Even if you’re small, you want people to see you as a professional business. If you’re still growing, you need the building blocks to get you where you want to be. I’ve learned so much about business through Google Workspace—I can’t imagine working without it. (Email us for more codes)
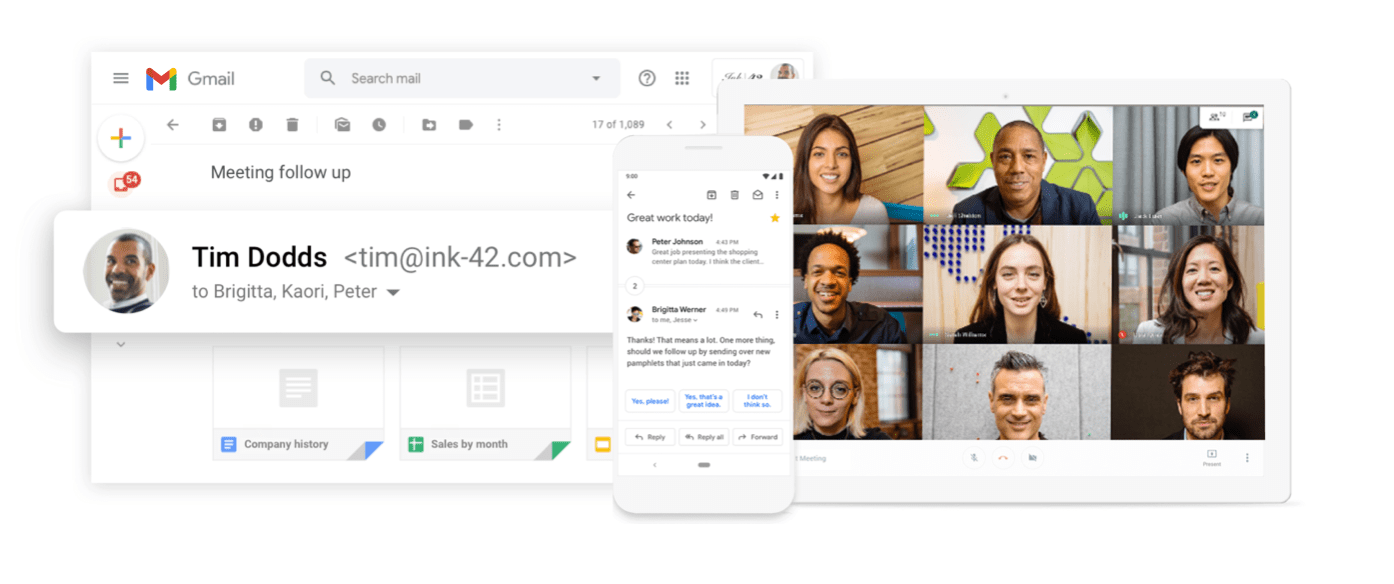
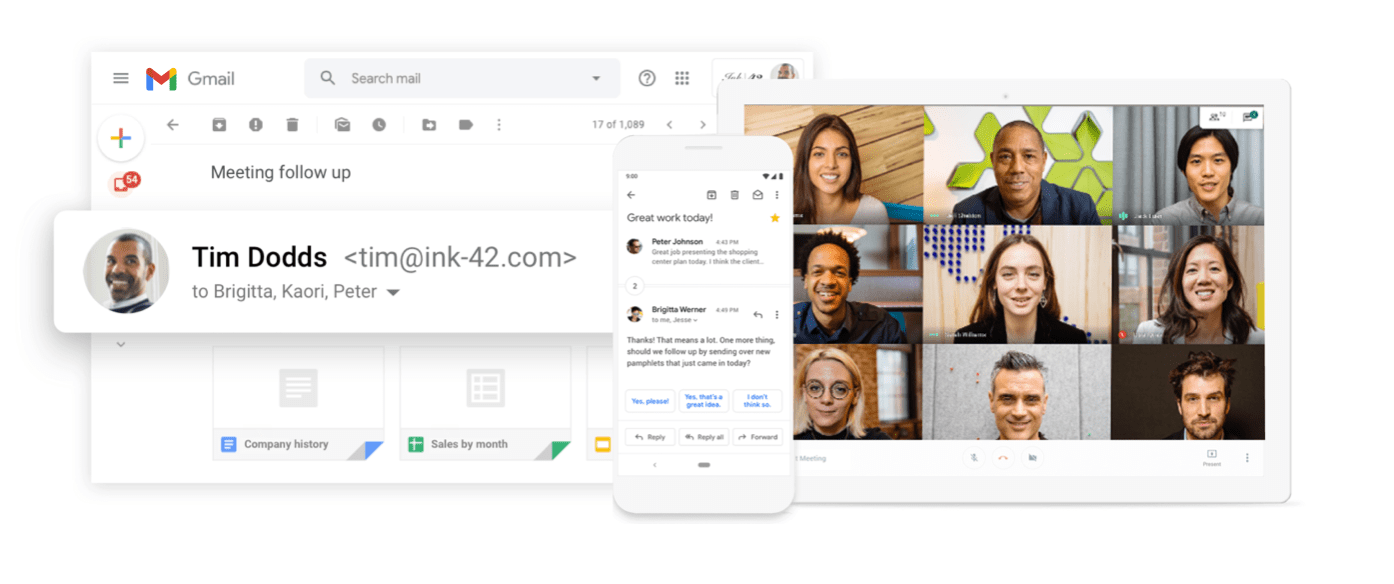