How to Get all All AWS Ec2 snapshot reports with python and boto3?
Use this python script to get all EC2 snapshot report in your AWS account.

import boto3
def lambda_handler(event, context):
session = boto3.Session(profile_name='saml')
ec2client = session.client('ec2')
instances_with_volumes = get_instance_ids(ec2client, "tc:OpsAutomatorTaskList")
for instance_id, volume_ids in instances_with_volumes.items():
response = ec2client.describe_snapshots(
Filters = [
{'Name':'volume-id', 'Values': volume_ids}
#{'Name':'start-time', 'Values': ['2019-01-01']}
]
#MaxResults = 10
)
#print(response['ResponseMetadata'])
#print("instance_id:%s, number_of_snapshots:%s" % (instance_id, len(response['Snapshots'])))
if len(response['Snapshots']) > 0:
#print(response['Tags'])
#Print Header
print('InstanceId' + ' , ' + 'Description' + ' , ' + 'Encrypted' + ' , ' + 'OwnerId' + ' , ' + 'Progress' + ' , ' + 'SnapshotId' + ' , ' + 'StartTime' + ' , ' + 'State' + ' , ' + 'VolumeId' + ' , ' + 'VolumeSize')
for snapshot in response['Snapshots']:
print(instance_id + ' , ' + snapshot['Description'] + ' , ' + str(snapshot['Encrypted']) + ' , ' + str(snapshot['OwnerId']) + ' , ' + snapshot['Progress'] + ' , ' + snapshot['SnapshotId'] + ' , ' + str(snapshot['StartTime']) + ' , ' + snapshot['State'] + ' , ' + snapshot['VolumeId'] + ' , ' + str(snapshot['VolumeSize']) )
def get_instance_ids(ec2client, tag_name):
# When passed a tag key, tag value this will return a list of InstanceIds that were found.
response = ec2client.describe_instances(
Filters=[
{
‘Name’: ‘tag-key’,
‘Values’: [tag_name]
}
]
)
instancelist = {}
for reservation in (response[“Reservations”]):
for instance in reservation[“Instances”]:
ebs_volume_ids = []
for ebs in instance[‘BlockDeviceMappings’]:
ebs_volume_ids.append(ebs[‘Ebs’][‘VolumeId’])
instancelist[instance[“InstanceId”]] = ebs_volume_ids
return instancelist
Advertise with us - Post Your Good Content Here
We are ranked in the Top 20 on Google
AI Dashboard is available on the Web, Apple, Google, and Microsoft, PRO version
lambda_handler(”,”)
Active Hydrating Toner, Anti-Aging Replenishing Advanced Face Moisturizer, with Vitamins A, C, E & Natural Botanicals to Promote Skin Balance & Collagen Production, 6.7 Fl Oz
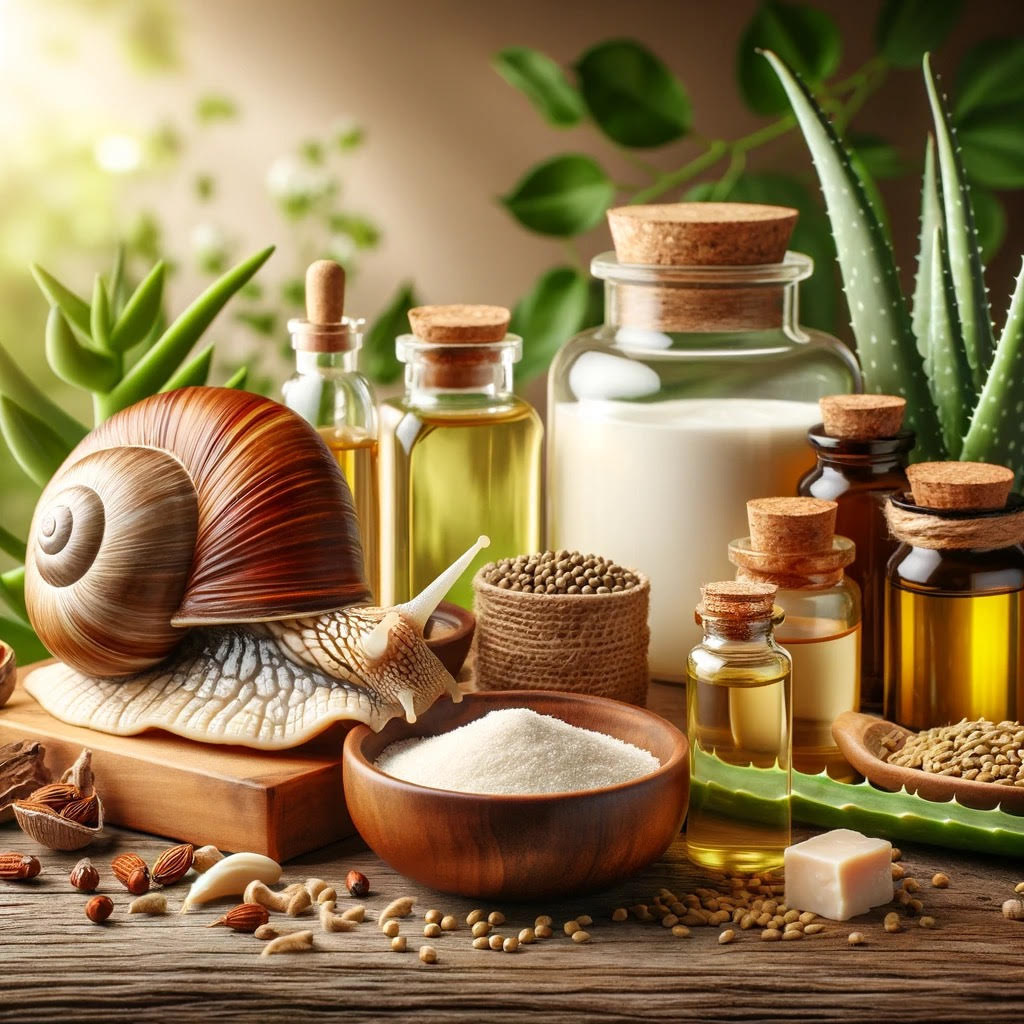

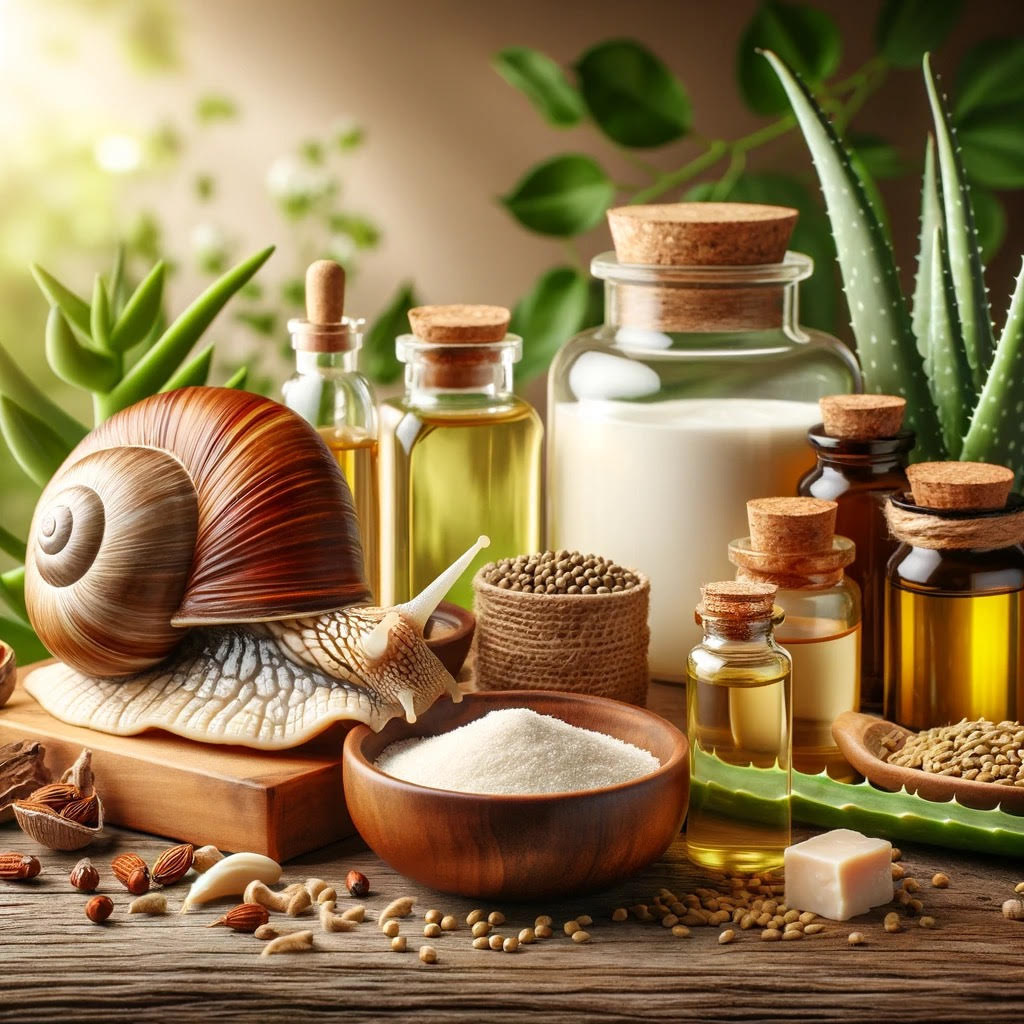

Age Defying 0.3% Retinol Serum, Anti-Aging Dark Spot Remover for Face, Fine Lines & Wrinkle Pore Minimizer, with Vitamin E & Natural Botanicals
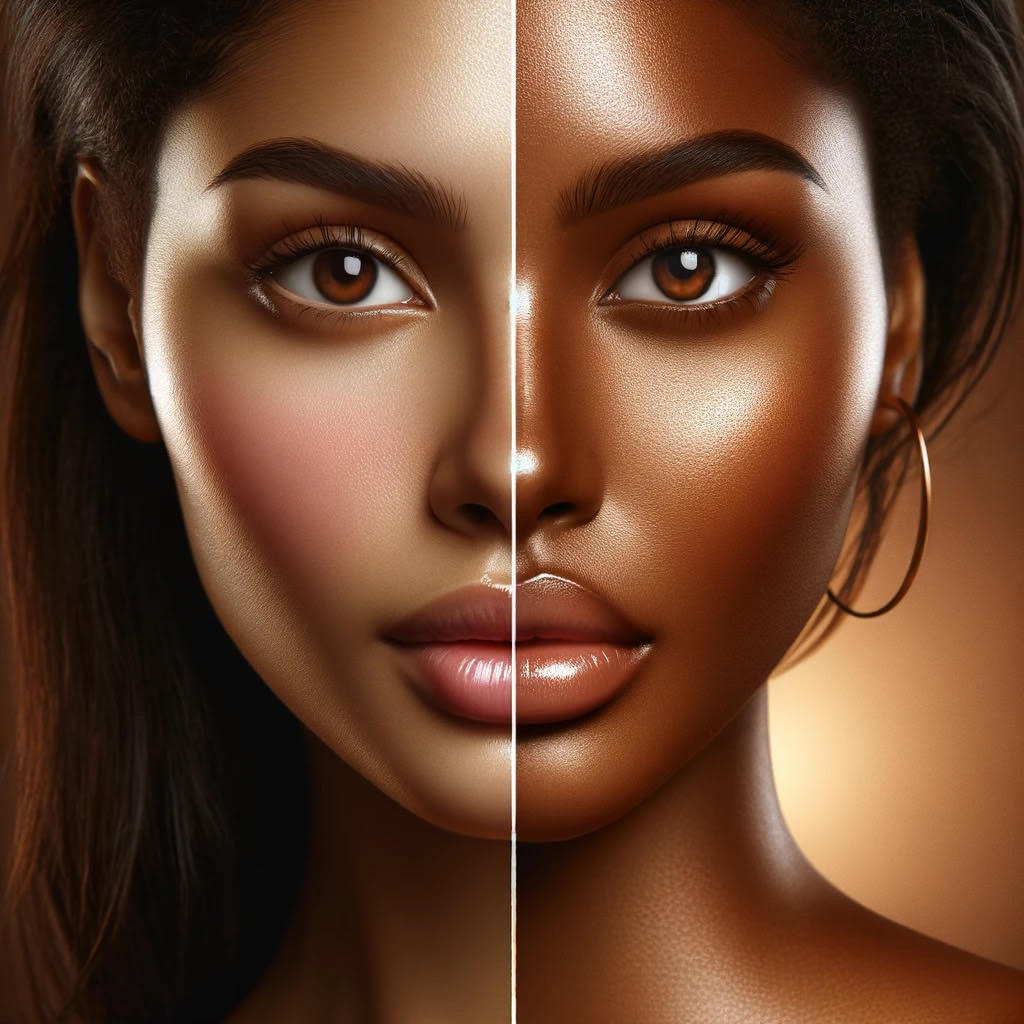

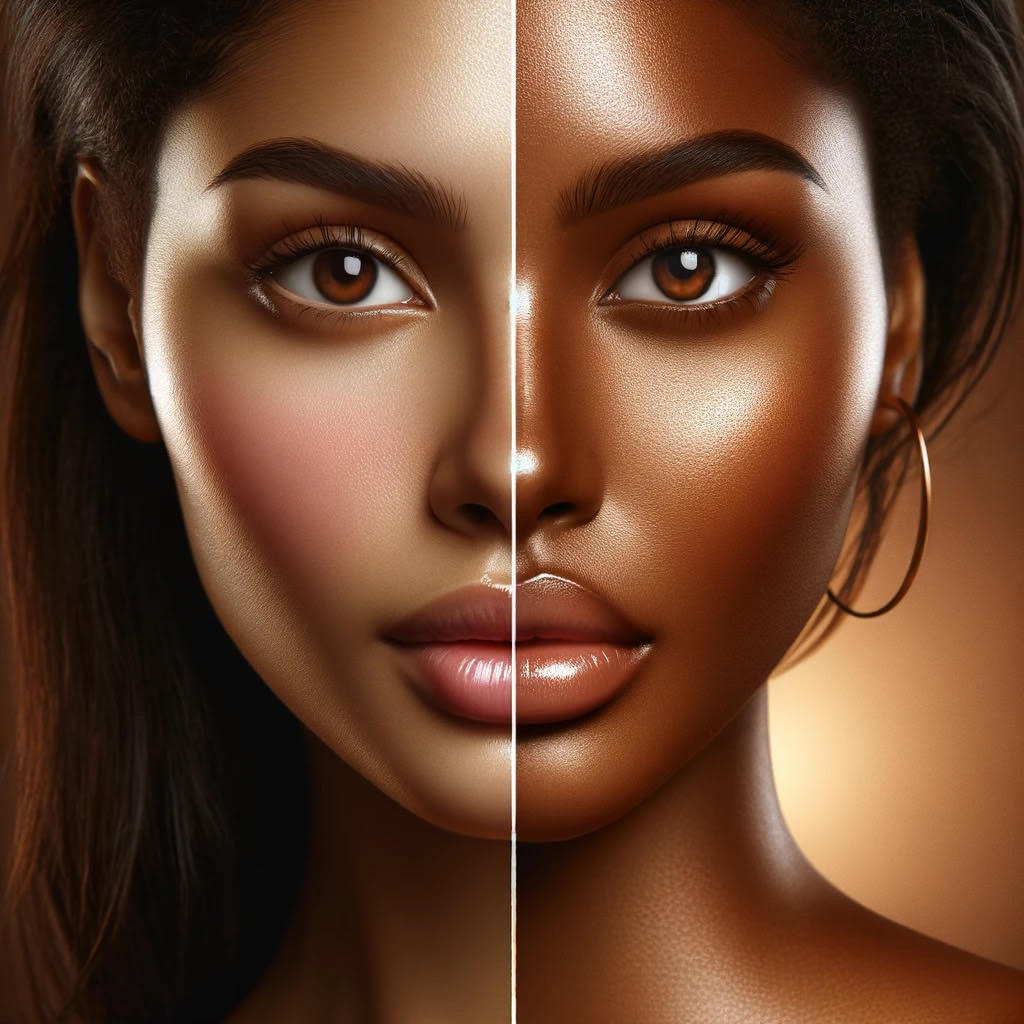

Firming Moisturizer, Advanced Hydrating Facial Replenishing Cream, with Hyaluronic Acid, Resveratrol & Natural Botanicals to Restore Skin's Strength, Radiance, and Resilience, 1.75 Oz


Skin Stem Cell Serum
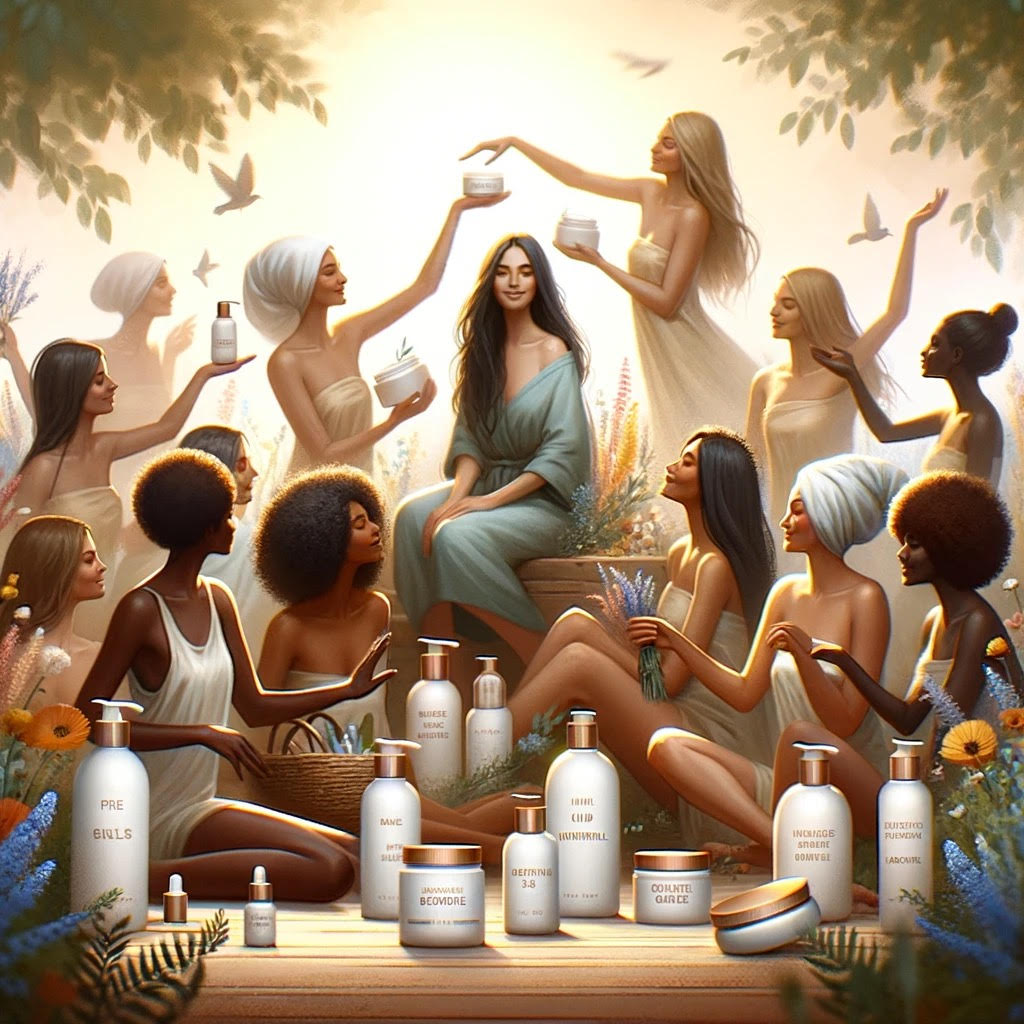

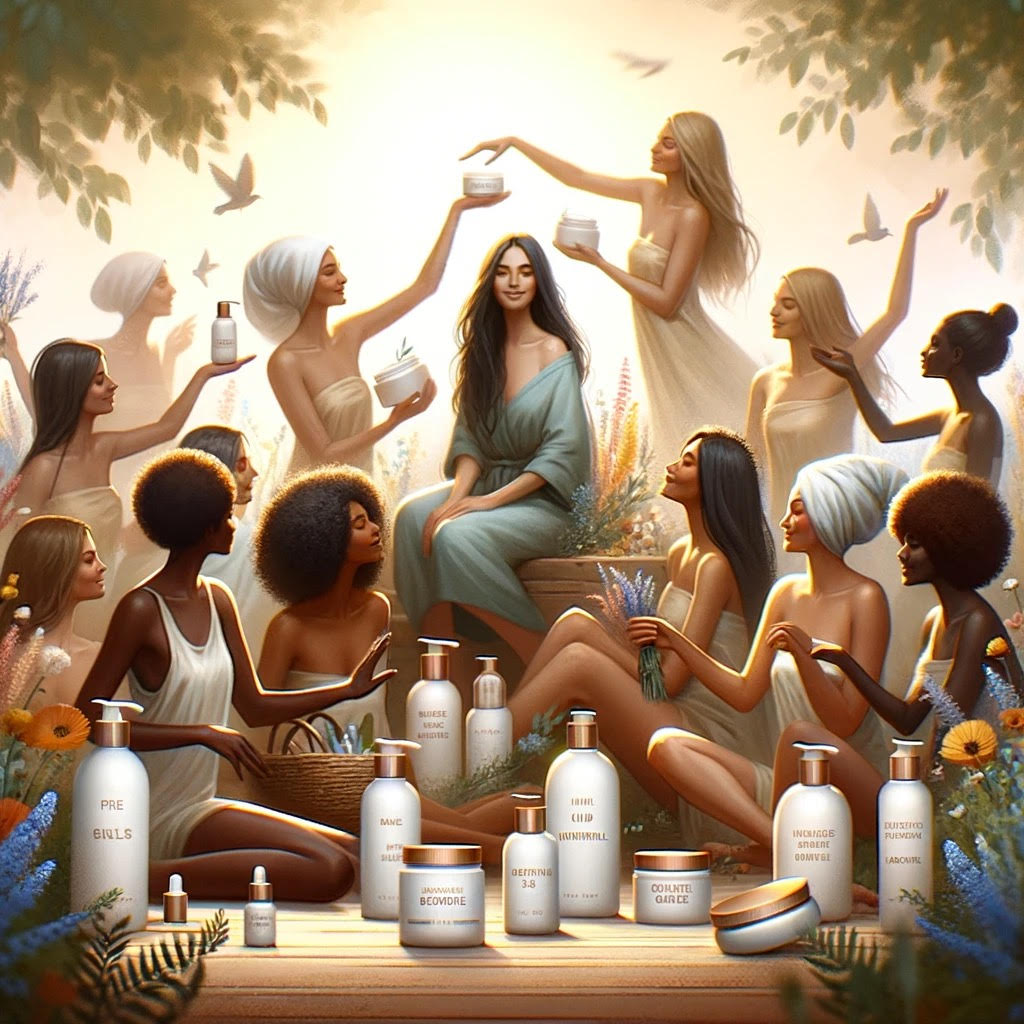

Smartphone 101 - Pick a smartphone for me - android or iOS - Apple iPhone or Samsung Galaxy or Huawei or Xaomi or Google Pixel
Can AI Really Predict Lottery Results? We Asked an Expert.
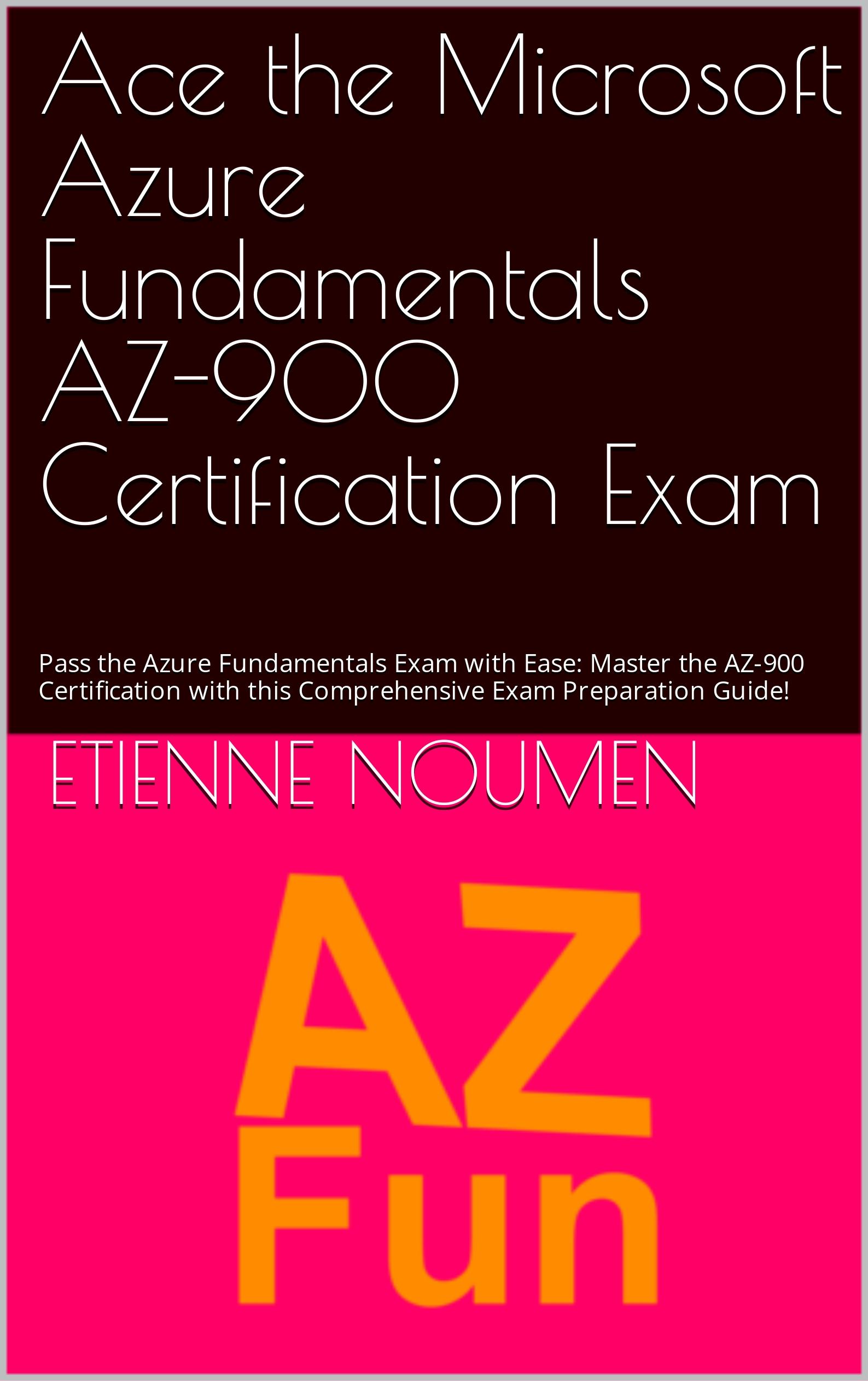
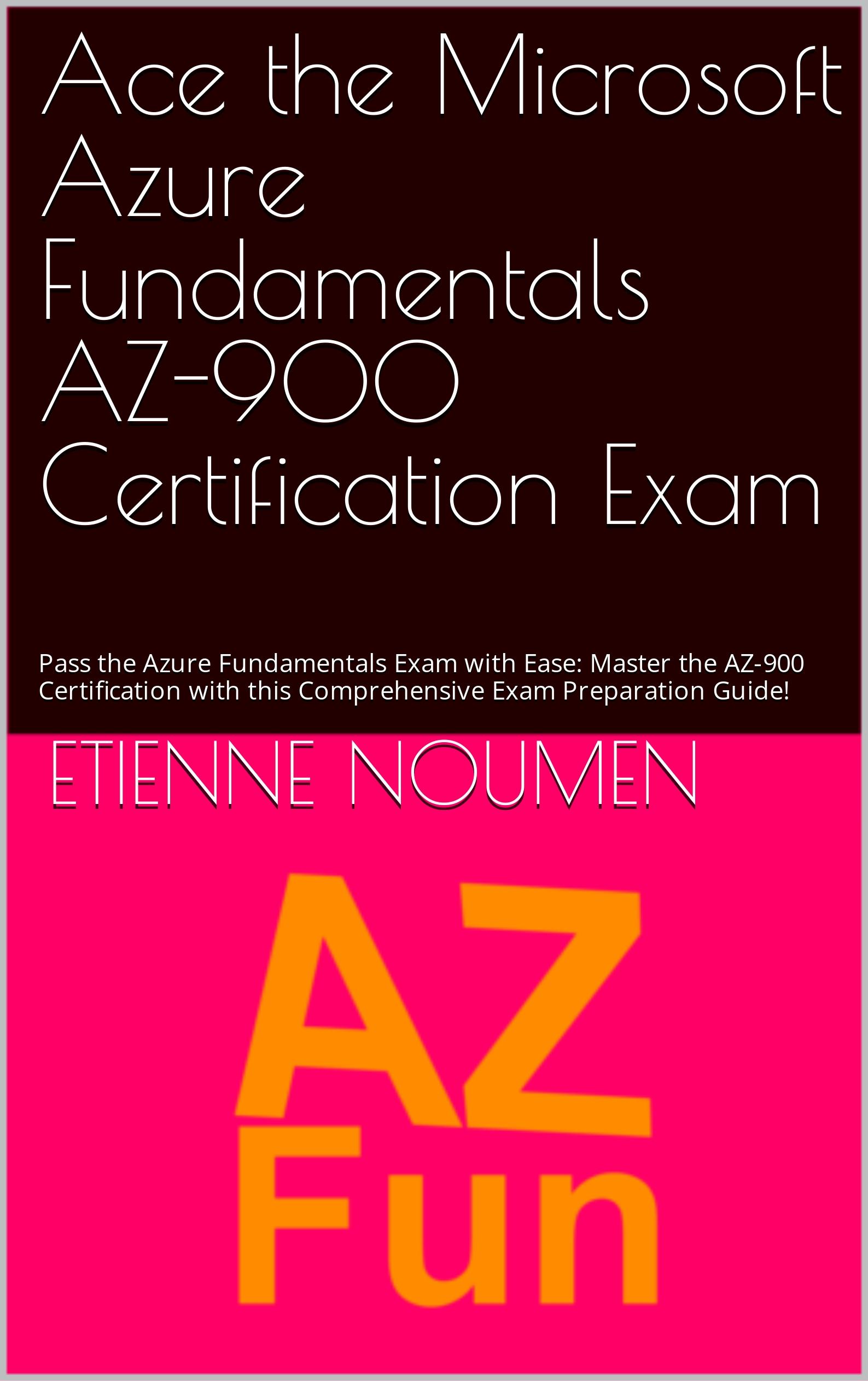


Djamgatech


Read Photos and PDFs Aloud for me iOS
Read Photos and PDFs Aloud for me android
Read Photos and PDFs Aloud For me Windows 10/11
Read Photos and PDFs Aloud For Amazon

Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE (Email us for more)
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes: 96DRHDRA9J7GTN6(Email us for more)

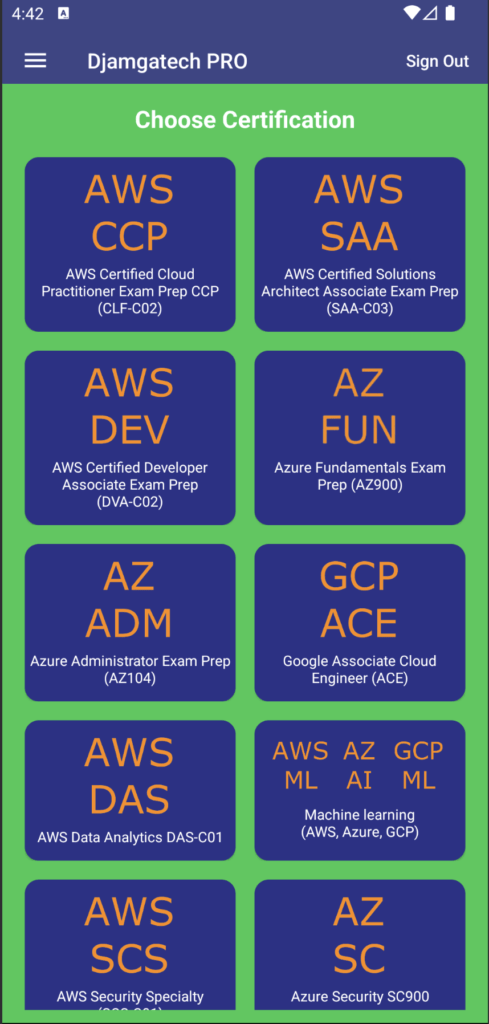
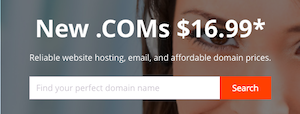
FREE 10000+ Quiz Trivia and and Brain Teasers for All Topics including Cloud Computing, General Knowledge, History, Television, Music, Art, Science, Movies, Films, US History, Soccer Football, World Cup, Data Science, Machine Learning, Geography, etc....
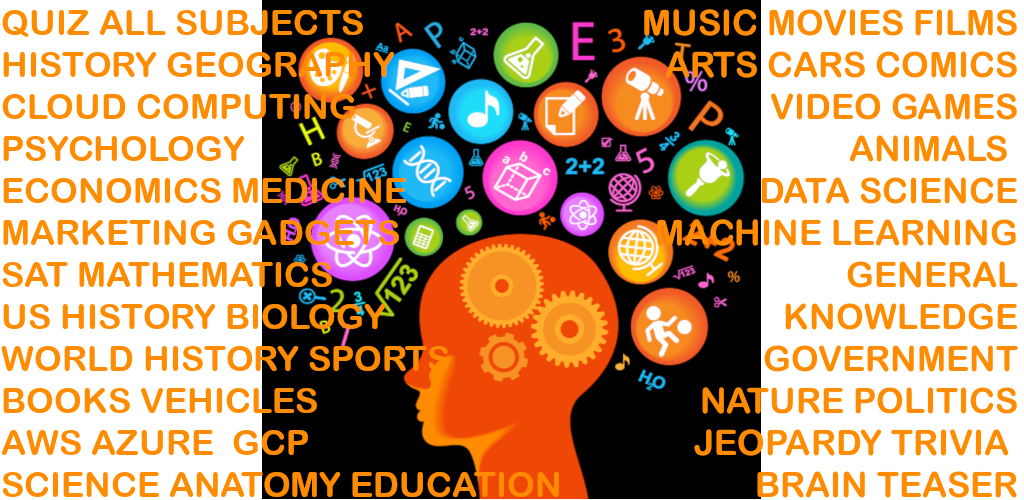

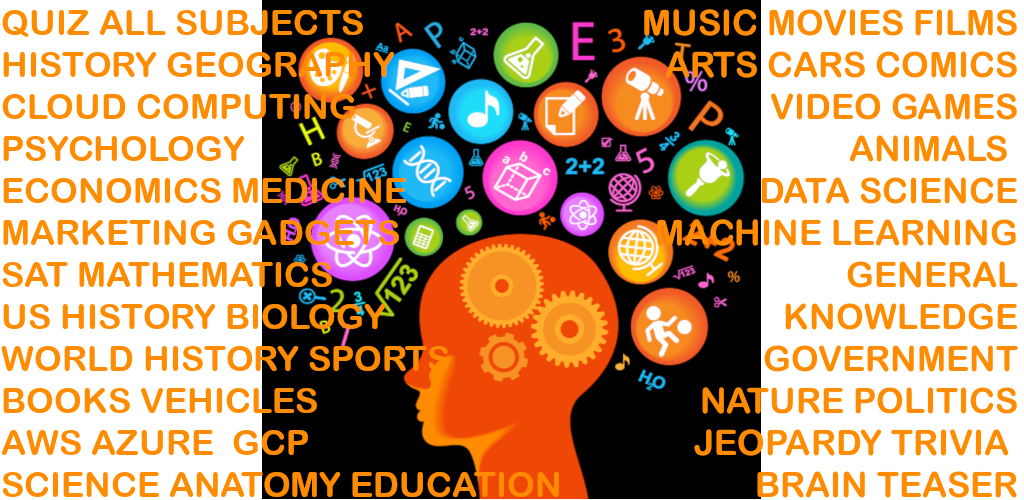
List of Freely available programming books - What is the single most influential book every Programmers should read
- Bjarne Stroustrup - The C++ Programming Language
- Brian W. Kernighan, Rob Pike - The Practice of Programming
- Donald Knuth - The Art of Computer Programming
- Ellen Ullman - Close to the Machine
- Ellis Horowitz - Fundamentals of Computer Algorithms
- Eric Raymond - The Art of Unix Programming
- Gerald M. Weinberg - The Psychology of Computer Programming
- James Gosling - The Java Programming Language
- Joel Spolsky - The Best Software Writing I
- Keith Curtis - After the Software Wars
- Richard M. Stallman - Free Software, Free Society
- Richard P. Gabriel - Patterns of Software
- Richard P. Gabriel - Innovation Happens Elsewhere
- Code Complete (2nd edition) by Steve McConnell
- The Pragmatic Programmer
- Structure and Interpretation of Computer Programs
- The C Programming Language by Kernighan and Ritchie
- Introduction to Algorithms by Cormen, Leiserson, Rivest & Stein
- Design Patterns by the Gang of Four
- Refactoring: Improving the Design of Existing Code
- The Mythical Man Month
- The Art of Computer Programming by Donald Knuth
- Compilers: Principles, Techniques and Tools by Alfred V. Aho, Ravi Sethi and Jeffrey D. Ullman
- Gödel, Escher, Bach by Douglas Hofstadter
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
- Effective C++
- More Effective C++
- CODE by Charles Petzold
- Programming Pearls by Jon Bentley
- Working Effectively with Legacy Code by Michael C. Feathers
- Peopleware by Demarco and Lister
- Coders at Work by Peter Seibel
- Surely You're Joking, Mr. Feynman!
- Effective Java 2nd edition
- Patterns of Enterprise Application Architecture by Martin Fowler
- The Little Schemer
- The Seasoned Schemer
- Why's (Poignant) Guide to Ruby
- The Inmates Are Running The Asylum: Why High Tech Products Drive Us Crazy and How to Restore the Sanity
- The Art of Unix Programming
- Test-Driven Development: By Example by Kent Beck
- Practices of an Agile Developer
- Don't Make Me Think
- Agile Software Development, Principles, Patterns, and Practices by Robert C. Martin
- Domain Driven Designs by Eric Evans
- The Design of Everyday Things by Donald Norman
- Modern C++ Design by Andrei Alexandrescu
- Best Software Writing I by Joel Spolsky
- The Practice of Programming by Kernighan and Pike
- Pragmatic Thinking and Learning: Refactor Your Wetware by Andy Hunt
- Software Estimation: Demystifying the Black Art by Steve McConnel
- The Passionate Programmer (My Job Went To India) by Chad Fowler
- Hackers: Heroes of the Computer Revolution
- Algorithms + Data Structures = Programs
- Writing Solid Code
- JavaScript - The Good Parts
- Getting Real by 37 Signals
- Foundations of Programming by Karl Seguin
- Computer Graphics: Principles and Practice in C (2nd Edition)
- Thinking in Java by Bruce Eckel
- The Elements of Computing Systems
- Refactoring to Patterns by Joshua Kerievsky
- Modern Operating Systems by Andrew S. Tanenbaum
- The Annotated Turing
- Things That Make Us Smart by Donald Norman
- The Timeless Way of Building by Christopher Alexander
- The Deadline: A Novel About Project Management by Tom DeMarco
- The C++ Programming Language (3rd edition) by Stroustrup
- Patterns of Enterprise Application Architecture
- Computer Systems - A Programmer's Perspective
- Agile Principles, Patterns, and Practices in C# by Robert C. Martin
- Growing Object-Oriented Software, Guided by Tests
- Framework Design Guidelines by Brad Abrams
- Object Thinking by Dr. David West
- Advanced Programming in the UNIX Environment by W. Richard Stevens
- Hackers and Painters: Big Ideas from the Computer Age
- The Soul of a New Machine by Tracy Kidder
- CLR via C# by Jeffrey Richter
- The Timeless Way of Building by Christopher Alexander
- Design Patterns in C# by Steve Metsker
- Alice in Wonderland by Lewis Carol
- Zen and the Art of Motorcycle Maintenance by Robert M. Pirsig
- About Face - The Essentials of Interaction Design
- Here Comes Everybody: The Power of Organizing Without Organizations by Clay Shirky
- The Tao of Programming
- Computational Beauty of Nature
- Writing Solid Code by Steve Maguire
- Philip and Alex's Guide to Web Publishing
- Object-Oriented Analysis and Design with Applications by Grady Booch
- Effective Java by Joshua Bloch
- Computability by N. J. Cutland
- Masterminds of Programming
- The Tao Te Ching
- The Productive Programmer
- The Art of Deception by Kevin Mitnick
- The Career Programmer: Guerilla Tactics for an Imperfect World by Christopher Duncan
- Paradigms of Artificial Intelligence Programming: Case studies in Common Lisp
- Masters of Doom
- Pragmatic Unit Testing in C# with NUnit by Andy Hunt and Dave Thomas with Matt Hargett
- How To Solve It by George Polya
- The Alchemist by Paulo Coelho
- Smalltalk-80: The Language and its Implementation
- Writing Secure Code (2nd Edition) by Michael Howard
- Introduction to Functional Programming by Philip Wadler and Richard Bird
- No Bugs! by David Thielen
- Rework by Jason Freid and DHH
- JUnit in Action
#BlackOwned #BlackEntrepreneurs #BlackBuniness #AWSCertified #AWSCloudPractitioner #AWSCertification #AWSCLFC02 #CloudComputing #AWSStudyGuide #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AWSBasics #AWSCertified #AWSMachineLearning #AWSCertification #AWSSpecialty #MachineLearning #AWSStudyGuide #CloudComputing #DataScience #AWSCertified #AWSSolutionsArchitect #AWSArchitectAssociate #AWSCertification #AWSStudyGuide #CloudComputing #AWSArchitecture #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AzureFundamentals #AZ900 #MicrosoftAzure #ITCertification #CertificationPrep #StudyMaterials #TechLearning #MicrosoftCertified #AzureCertification #TechBooks
Top 1000 Canada Quiz and trivia: CANADA CITIZENSHIP TEST- HISTORY - GEOGRAPHY - GOVERNMENT- CULTURE - PEOPLE - LANGUAGES - TRAVEL - WILDLIFE - HOCKEY - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION



Top 1000 Africa Quiz and trivia: HISTORY - GEOGRAPHY - WILDLIFE - CULTURE - PEOPLE - LANGUAGES - TRAVEL - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION
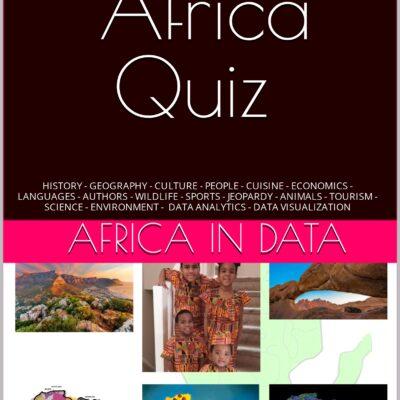

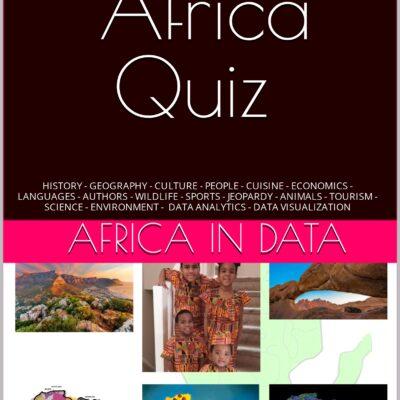
Exploring the Pros and Cons of Visiting All Provinces and Territories in Canada.



Exploring the Advantages and Disadvantages of Visiting All 50 States in the USA



Health Health, a science-based community to discuss health news and the coronavirus (COVID-19) pandemic
- Fentanyl’s deadly chemistry: How criminals make illicit opioidsby /u/Maxcactus on July 26, 2024 at 8:14 am
submitted by /u/Maxcactus [link] [comments]
- A New Artificial Heart in Houston Might Save Millionsby /u/zsreport on July 25, 2024 at 11:13 pm
submitted by /u/zsreport [link] [comments]
- Medical boards punished these doctors and nurses. South Carolina prisons hired them anyway.by /u/jms1225 on July 25, 2024 at 8:06 pm
submitted by /u/jms1225 [link] [comments]
- US infant mortality increased in 2022 for the first time in decades, CDC report showsby /u/cnn on July 25, 2024 at 6:37 pm
submitted by /u/cnn [link] [comments]
- Study raises hopes that shingles vaccine may delay onset of dementia | Dementia | The Guardianby /u/chilladipa on July 25, 2024 at 3:38 pm
submitted by /u/chilladipa [link] [comments]
Today I Learned (TIL) You learn something new every day; what did you learn today? Submit interesting and specific facts about something that you just found out here.
- TIL that George Takei respectfully declined an offer to voice himself in the Simpsons episode Marge vs. the Monorail because he didn't want to ridicule public transportationby /u/Lucy_loveebby on July 26, 2024 at 7:08 am
submitted by /u/Lucy_loveebby [link] [comments]
- TIL about Shizo Kanakuri, a Japanese runner who dropped out of the 1912 Olympic race (he fell asleep during his rest mid-race), but returned 54 years later to officially finish it. Kanakuri joked: “It was a long trip. Along the way, I got married, had six children and 10 grandchildren.”by /u/EnigmaticScience on July 26, 2024 at 6:57 am
submitted by /u/EnigmaticScience [link] [comments]
- TIL Gerhard Domagk’s discovery of the antibacterial properties of Prontosil won him the 1939 Nobel Prize in Medicine but German citizens were forbidden to accept the prize under Hitler. Domagk was forced to reject it by the Gespato before receiving it in 1947 but without the prize moneyby /u/ubcstaffer123 on July 26, 2024 at 3:56 am
submitted by /u/ubcstaffer123 [link] [comments]
- Today I learned that the actor Keegan-Michael Key had two biological half-siblings (one of whom was comic book writer Dwayne McDuffie) and Key didn't know about either of them until after both of them had died.by /u/wimpykidfan37 on July 26, 2024 at 3:31 am
submitted by /u/wimpykidfan37 [link] [comments]
- TIL that average human height went down from 5'10" (178 cm) for men and 5'6" (168 cm) for women to 5'5" (165 cm) and 5'1" (155 cm) 10,000 years ago and it took until the 20th century for average human height to match pre-Neolithic Revolution levels.by /u/quadrahex on July 26, 2024 at 2:04 am
submitted by /u/quadrahex [link] [comments]
Reddit Science This community is a place to share and discuss new scientific research. Read about the latest advances in astronomy, biology, medicine, physics, social science, and more. Find and submit new publications and popular science coverage of current research.
- New study uses Game of Thrones to advance understanding of face blindness. In people with prosopagnosia, the effect of familiarity was not found in the same regions of the brain as it was in neurotypical participants while watching the TV show. Face blindness affects approximately 1 in 50 people.by /u/mvea on July 26, 2024 at 7:45 am
submitted by /u/mvea [link] [comments]
- MIT scientists have discovered an intriguing new way to produce hydrogen fuel, using just soda cans, seawater and coffee grounds. The team says the chemical reaction could be put to work powering engines or fuel cells in marine vehicles like submarines that suck in seawater.by /u/mvea on July 26, 2024 at 7:37 am
submitted by /u/mvea [link] [comments]
- Science Impact: Experimental Warn-On-Forecast System Yields 75-Minute Lead Time on Violent Tornado {NOAA Press Release}by /u/Elijah-Joyce-Weather on July 26, 2024 at 4:55 am
submitted by /u/Elijah-Joyce-Weather [link] [comments]
- 65 million Americans now own firearms for protection, suggests the results of a nationally representative survey carried out in 2023by /u/FunnyGamer97 on July 26, 2024 at 2:16 am
submitted by /u/FunnyGamer97 [link] [comments]
- Examining tornado exposure, post-tornado distress, and gender following the March 2020 tornado in Nashville, Tennesseeby /u/Elijah-Joyce-Weather on July 25, 2024 at 11:49 pm
submitted by /u/Elijah-Joyce-Weather [link] [comments]
Reddit Sports Sports News and Highlights from the NFL, NBA, NHL, MLB, MLS, and leagues around the world.
- Canada's men's and women's soccer teams have relied on drones and spying for years, sources sayby /u/FireLychee on July 26, 2024 at 3:37 am
submitted by /u/FireLychee [link] [comments]
- After a grueling Tour de France, top riders are racing to recover for Paris Olympics time trialby /u/Oldtimer_2 on July 26, 2024 at 12:54 am
submitted by /u/Oldtimer_2 [link] [comments]
- Canada WNT coach Bev Priestman suspended, sent home from Olympics amid spying scandalby /u/Oldtimer_2 on July 26, 2024 at 12:49 am
submitted by /u/Oldtimer_2 [link] [comments]
- 'End of an Era': TNT's 'Inside the NBA' Ending After NBA Chooses Amazonby /u/lame_building14 on July 26, 2024 at 12:36 am
submitted by /u/lame_building14 [link] [comments]
- Canada axes coach from Olympics over drone useby /u/chief_sitass on July 26, 2024 at 12:31 am
submitted by /u/chief_sitass [link] [comments]
Turn your dream into reality with Google Workspace: It’s free for the first 14 days.
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes:
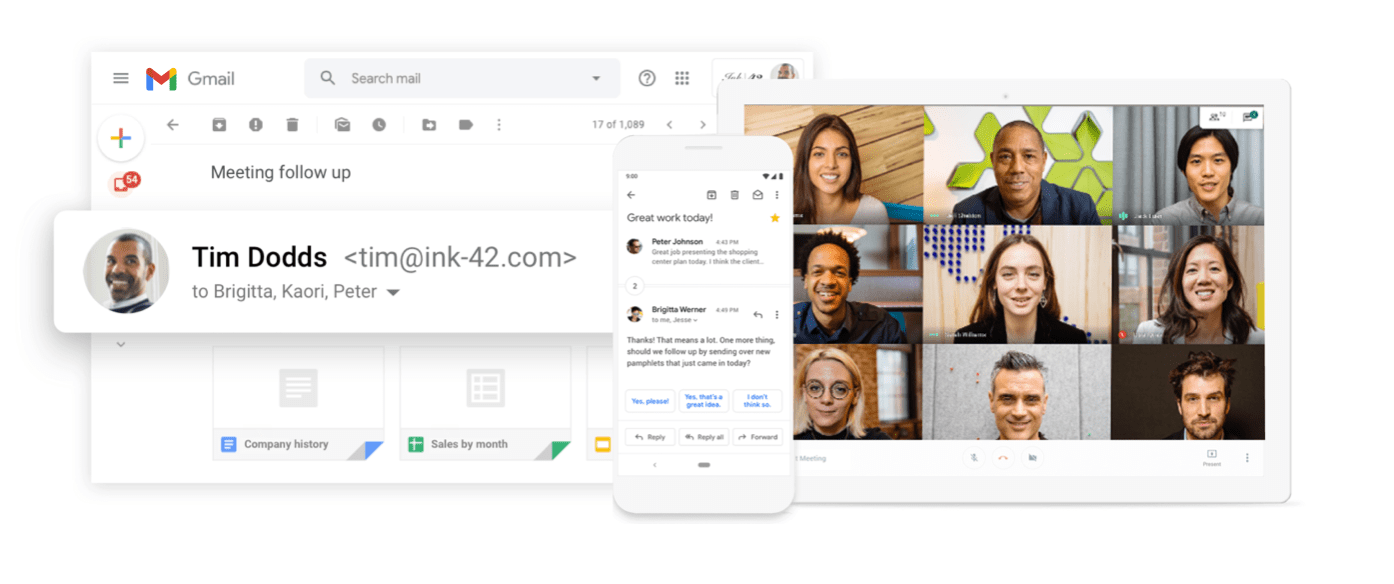

96DRHDRA9J7GTN6
63F733CLLY7R7MM
63F7D7CPD9XXUVT
63FLKQHWV3AEEE6
63JGLWWK36CP7WM
63KKR9EULQRR7VE
63KNY4N7VHCUA9R
63LDXXFYU6VXDG9
63MGNRCKXURAYWC
63NGNDVVXJP4N99
63P4G3ELRPADKQU
With Google Workspace, Get custom email @yourcompany, Work from anywhere; Easily scale up or down
Google gives you the tools you need to run your business like a pro. Set up custom email, share files securely online, video chat from any device, and more.
Google Workspace provides a platform, a common ground, for all our internal teams and operations to collaboratively support our primary business goal, which is to deliver quality information to our readers quickly.
Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE
C37HCAQRVR7JTFK
C3AE76E7WATCTL9
C3C3RGUF9VW6LXE
C3D9LD4L736CALC
C3EQXV674DQ6PXP
C3G9M3JEHXM3XC7
C3GGR3H4TRHUD7L
C3LVUVC3LHKUEQK
C3PVGM4CHHPMWLE
C3QHQ763LWGTW4C
Even if you’re small, you want people to see you as a professional business. If you’re still growing, you need the building blocks to get you where you want to be. I’ve learned so much about business through Google Workspace—I can’t imagine working without it. (Email us for more codes)
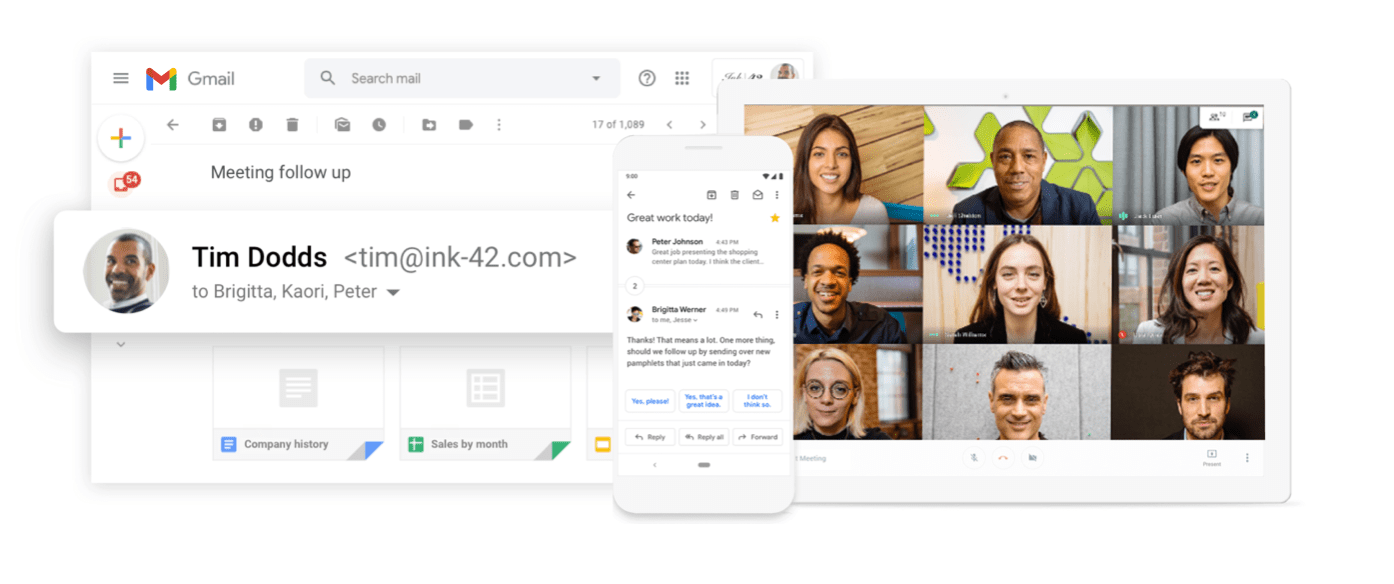
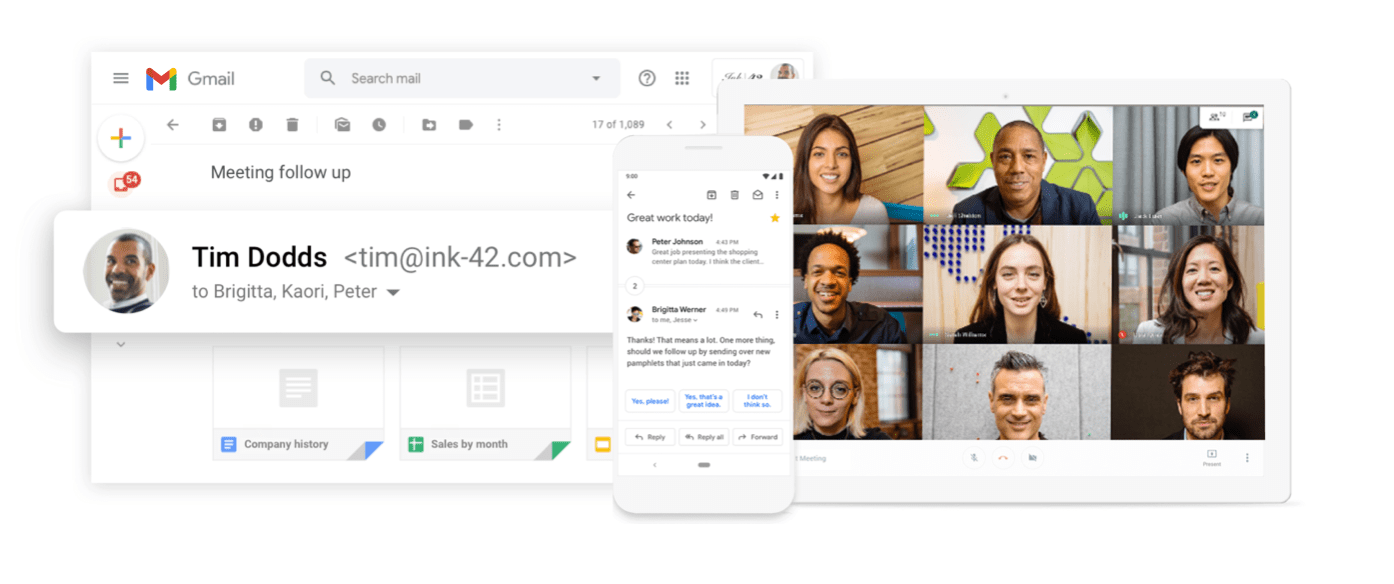