AI Dashboard is available on the Web, Apple, Google, and Microsoft, PRO version
How do you make a Python loop faster?
Programmers are always looking for ways to make their code more efficient. One way to do this is to use a faster loop. Python is a high-level programming language that is widely used by developers and software engineers. It is known for its readability and ease of use. However, one downside of Python is that its loops can be slow. This can be a problem when you need to process large amounts of data. There are several ways to make Python loops faster. One way is to use a faster looping construct, such as C. Another way is to use an optimized library, such as NumPy. Finally, you can vectorize your code, which means converting it into a format that can be run on a GPU or other parallel computing platform. By using these techniques, you can significantly speed up your Python code.
According to Vladislav Zorov, If not talking about NumPy or something, try to use list comprehension expressions where possible. Those are handled by the C code of the Python interpreter, instead of looping in Python. Basically same idea like the NumPy solution, you just don’t want code running in Python.
Example: (Python 3.0)

Python list traversing tip:
Instead of this: for i in range(len(l)): x = l[i]
Use this for i, x in enumerate(l): …
TO keep track of indices and values inside a loop.
Twice faster, and the code looks better.
Finally, developers can also improve the performance of their code by making use of caching. By caching values that are computed inside a loop, programmers can avoid having to recalculate them each time through the loop. By taking these steps, programmers can make their Python code more efficient and faster.
Very Important: Don’t worry about code efficiency until you find yourself needing to worry about code efficiency.
The place where you think about efficiency is within the logic of your implementations.
This is where “big O” discussions come in to play. If you aren’t familiar, here is a link on the topic
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes: 96DRHDRA9J7GTN6
Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE (Email us for more codes)
What are the top 10 Wonders of computing and software engineering?

Do you want to learn python we found 5 online coding courses for beginners?
Python Coding Bestsellers on Amazon
https://amzn.to/3s3KXc3
The Best Python Coding and Programming Bootcamps
We’ve also included a scholarship resource with more than 40 unique scholarships to provide additional financial support.
Python Coding Breaking News
- Stumbling my way throughby /u/-Doodoofard (Python) on May 3, 2024 at 9:22 pm
New to python and trying to create a simple calculator of my own design just wondering how I could get x to times input1 and input2 together (x is whatever is inputed)(intended to be either / or * or +/-) submitted by /u/-Doodoofard [link] [comments]
- Migrate data from one MongoDB cluster to another MongoDB cluster using Pythonby /u/vmanel96 (Python) on May 3, 2024 at 6:00 pm
I need to migrate around 1 billion records from one MongoDB cluster to another MongoDB cluster using Python. What's the best way to do it using Python. Basically I need to insert data in batches and batch size should be controllable, and multi threads/process should be running. Logging should be present, and if the script stops in between it should continue process from where it stopped. Exception handling and retries are required. Any other approach / libraries which would simplify this? submitted by /u/vmanel96 [link] [comments]
- New book! The Quick Python Book, Fourth Edition by Naomi Cederby /u/ManningBooks (Python) on May 3, 2024 at 1:42 pm
Hello everybody, Thank you for having us here, and a huge "Thank you" to the moderators for letting us post. We have just released the latest edition of The Quick Python Book by the one-and-only Naomi Ceder, and I wanted to share that news with the community. Many of you are already familiar with Naomi's work and her massive contributions to the world of Python programming language. The Quick Python Book has aided over 100,000 developers in mastering Python. The Fourth Edition of the book has been revised to include the latest features, control structures, and libraries of Python, along with new coverage of working with AI-generated Python code. Naomi, the author, has beautifully balanced the details of the language with the insights and advice required to accomplish any task. Her personal touch has made learning Python an enjoyable experience for countless developers. 📚 You can find the book here: https://mng.bz/aEQj 📖 Get into the liveBook: https://mng.bz/gvee And last but not the least, get 46% off with code: receder46 Hope you find the book helpful. Thank you. Cheers, submitted by /u/ManningBooks [link] [comments]
- Project: Simple Interactive Python Streamlit Maps With NASA GIS Databy /u/jgloewen (Python) on May 3, 2024 at 10:22 am
Python Streamlit is terrific for putting together interactive dashboards. Combined with the geopandas library, streamlit can easily display GIS data points on a map for you. Forest fires in my home province of British Columbia, Canada have been really bad recently. NASA has a terrific dataset that keeps track of forest fires by country. Can I use Streamlit to access this dataset and display a map off all the fires within a certain area (BC) for a particular time frame (2021)? And can I give the user the ability to choose a month? You bet! Let me step you through how! FREE tutorial (with code): https://johnloewen.substack.com/p/simple-interactive-python-streamlit submitted by /u/jgloewen [link] [comments]
- typedattr: Autocompletion and typechecking for CLI script arguments, using standard argparse syntaxby /u/gings7 (Python) on May 3, 2024 at 7:45 am
Excited to share my pypi package typedparser I have been working on for around 1 year now. What My Project Does: It enables writing CLI scripts and create an "args" variable with autocompleted members and type checks, but still keeps the simple and universally understood syntax of the stdlib argarse module. Target Audience: For stability, I battletested it in my research projects and added automatic builds as well as 80%+ test coverage. So I believe it is pretty stable. Comparison: For typing functionality it uses the attrs package as backend. It also provides some additional features for object and dictionary manipulation. Of course there are many other CLI argument packages out there, but this one stands out in that it tries to keep the syntax of the argparse standard library as much as possible, making it easy for others to figure out what your script does. Check it out and let me know what you think. submitted by /u/gings7 [link] [comments]
- Friday Daily Thread: r/Python Meta and Free-Talk Fridaysby /u/AutoModerator (Python) on May 3, 2024 at 12:00 am
Weekly Thread: Meta Discussions and Free Talk Friday 🎙️ Welcome to Free Talk Friday on /r/Python! This is the place to discuss the r/Python community (meta discussions), Python news, projects, or anything else Python-related! How it Works: Open Mic: Share your thoughts, questions, or anything you'd like related to Python or the community. Community Pulse: Discuss what you feel is working well or what could be improved in the /r/python community. News & Updates: Keep up-to-date with the latest in Python and share any news you find interesting. Guidelines: All topics should be related to Python or the /r/python community. Be respectful and follow Reddit's Code of Conduct. Example Topics: New Python Release: What do you think about the new features in Python 3.11? Community Events: Any Python meetups or webinars coming up? Learning Resources: Found a great Python tutorial? Share it here! Job Market: How has Python impacted your career? Hot Takes: Got a controversial Python opinion? Let's hear it! Community Ideas: Something you'd like to see us do? tell us. Let's keep the conversation going. Happy discussing! 🌟 submitted by /u/AutoModerator [link] [comments]
- Dash vs Reflex vs Othersby /u/Sea_Split_1182 (Python) on May 2, 2024 at 10:56 pm
Where can I find a decent comparison (pros and cons) of these 5 solutions? They seem to be solving the same problem, which is, afaiu, separating the frontend ‘annoyance’ from Python scripting / math. Reflex (used to be called Pynecone) https://reflex.dev Streamlit https://streamlit.io Gradio https://gradio.app Dash https://dash.plotly.com Panel https://panel.holoviz.org/ Anvil https://anvil.works/ Quarto My use case: user access the web app, choose some parameters, selects things that go or not into a model. Python returns results of my math. Needs to be somewhat eye-candy and I need to use a lot of pictures to get the user input (i.e. “which of these figures you like most? 1,2,3. User clicks on “3”, 3 is considered in the model. submitted by /u/Sea_Split_1182 [link] [comments]
- I made a python package that can parse Excel Formula Strings into dictionary structures!by /u/MPGaming9000 (Python) on May 2, 2024 at 8:19 pm
What my project does: It basically takes a formula string like you'd get from Openpyxl like "=SUM(A1:B2)" and breaks it all out into a dictionary structure for you to then navigate through, modify, and then reformat that modified structure back into an excel friendly formula string again! Target Audience: (People who modify Excel formula strings in automated spreadsheet modification scripts. Or people who need to analyze formulas in a spreadsheet to do some kind of logic based on that analysis). Disclaimer: For most people some simple regex pattern matching and str replaces would be fine to modify formulas but if you need a more structured approach to working with these strings, this package has you covered! How does it differ compared to other projects: There are libraries like Openpyxl that allow you to tokenize and translate formulas but that's currently where it ends. It doesn't allow you to systematically parse out a formula and replace those pieces and add new structures and what not into it. Currently the best you can really do is translate formulas and anything other than that would need to rely on regex string matching logic or string replacements. (Which still would be fine for most people, but this just adds another layer of organization and scalability to the format). More info about it here: https://github.com/Voltaic314/ExcelFormulaParser To install, just do: pip install ExcelFormulaParser Thank you for reading this!! Hope you guys find it useful if you're ever systematically modifying (or analyzing) spreadsheets! submitted by /u/MPGaming9000 [link] [comments]
- Tutorial on Building a Server-to-Server Zoom App with Pythonby /u/SleekEagle (Python) on May 2, 2024 at 6:57 pm
I made a tutorial on how to build a server-to-server Zoom OAuth application using Python. This application can transcribe Zoom meeting recordings, print the transcripts to the terminal, and save the transcripts as text files. video tutorial repo written tutorial This tutorial covers: Setting up OAuth authentication for server-to-server apps Utilizing the Zoom API to access recordings Implementing automatic transcription using Python submitted by /u/SleekEagle [link] [comments]
- Starter Code for a LLM-based AI Assistantby /u/2bytesgoat (Python) on May 2, 2024 at 6:52 pm
Hey everyone 👋 TL;DR Since everyone is talking about the Humane AI Pin and the Rabbit R1, I decided to make a short 5 minute tutorial on how people can setup and customize their own little AI assistant on their machine. I've uploaded a video tutorial here: https://www.youtube.com/watch?v=2fD_SAouoOs&ab_channel=2BytesGoat And the Github code is here: https://github.com/2BYTESGOAT/AI-ASSISTANT Longer version What my project does: It's the starter code for an AI assistant that you can run locally. More precisely, it's a ChatGPT / Llama 2 agent that has access to Google Search and can get businesses nearby based on your location. The tool can be easily extended to support other APIs. Target audience: Pythoneers that are curious about LLMs and LLM related libraries. Comparison: It was inspired by projects such as the Humane AI Pin and the Rabbit R1. Though it's a inferior version to those, it serves more as a playground for people to develop their own AI assistants. submitted by /u/2bytesgoat [link] [comments]
- k8sAI - my open-source GPT CLI tool for Kubernetes!by /u/Wild_Plantain528 (Python) on May 2, 2024 at 3:52 pm
What my project does: I wanted to share an open-source project I’ve been working on called k8sAI. It’s a personal AI Kubernetes expert that can answer questions about your cluster, suggests commands, and even executes relevant kubectl commands to help diagnose and suggest fixes to your cluster, all in the CLI! Target Audience: As a relative newcomer to k8s, this tool has really streamlined my workflow. I can ask questions about my cluster, k8sAI will run kubectl commands to gather info, and then answer those question. It’s also found several issues in my cluster for me - all I’ve had to do is point it in the right direction. I’ve really enjoyed making and using this so I thought it could be useful for others. Added bonus is that you don’t need to copy and paste into ChatGPT anymore! k8sAI operates with read-only kubectl commands to make sure your cluster stays safe. All you need is an OpenAI API key and a valid kubectl config. Start chatting with k8sAI using: $ pip install k8sAI $ k8sAI chat or to fix an issue: $ k8sAI fix -p="take a look at the failing pod in the test namespace" Would love to get any feedback you guys have! Here's the repo for anyone who wants to take a look Comparison: I found a tool (k8sGPT) that I enjoyed using, but I felt it was still missing a few pieces on the chatbot side. You can't chat back and forth with k8sGPT and it doesn't suggest commands for you to execute, so I decided to make this. submitted by /u/Wild_Plantain528 [link] [comments]
- Multipart File Uploads to S3 with Pythonby /u/tylersavery (Python) on May 2, 2024 at 3:28 pm
I created this tutorial after overcoming a difficult challenge myself: uploading 5GB+ files to AWS. This approach allows the browser to securely upload directly to an S3 bucket without the file having to travel through the backend server. The implementation is written in python (backend) and vanilla js (frontend). submitted by /u/tylersavery [link] [comments]
- Hatch v1.10.0 - UV support, new test command and built-in script runnerby /u/Ofekmeister (Python) on May 2, 2024 at 2:00 pm
Hello everyone! I'd like to announce version 1.10.0: https://hatch.pypa.io/latest/blog/2024/05/02/hatch-v1100/ Feel free to provide any feedback either here or as a discussion on the repo: https://github.com/pypa/hatch submitted by /u/Ofekmeister [link] [comments]
- The Python on Microcontrollers (and Raspberry Pi) Newsletter, a weekly news and project resourceby /u/HP7933 (Python) on May 2, 2024 at 2:00 pm
The Python on Microcontrollers (and Raspberry Pi) Newsletter: subscribe for free With the Python on Microcontrollers newsletter, you get all the latest information on Python running on hardware in one place! MicroPython, CircuitPython and Python on single Board Computers like Raspberry Pi & many more. The Python on Microcontrollers newsletter is the place for the latest news. It arrives Monday morning with all the week’s happenings. No advertising, no spam, easy to unsubscribe. 10,958 subscribers - the largest Python on hardware newsletter out there. Catch all the weekly news on Python for Microcontrollers with adafruitdaily.com. This ad-free, spam-free weekly email is filled with CircuitPython, MicroPython, and Python information that you may have missed, all in one place! Ensure you catch the weekly Python on Hardware roundup– you can cancel anytime – try our spam-free newsletter today! https://www.adafruitdaily.com/ submitted by /u/HP7933 [link] [comments]
- What does your python development setup look like?by /u/Working_Noise_6043 (Python) on May 2, 2024 at 9:18 am
I'd like to explore other people's setup and perhaps try need things or extra tools. What kind IDE, any extra tools to make it easier for you, etc. Looking forward to everyone's responses! submitted by /u/Working_Noise_6043 [link] [comments]
- Suggestions for a self-hosted authentication as a service?by /u/FlyingRaijinEX (Python) on May 2, 2024 at 7:18 am
I have a simple backend REST API service that is serving a few ML models. I have made it "secured" by implementing an API key in order call those endpoints. I was wondering, how common it is for people to use services that can be self-hosted as their authentication/authorization. If it is common and reliable, what are the best options to go for? I've read that building your own authentication/authorization service with email verification, password reset, and social auth can be a pain. Also, did some googling and found this General - Fief. Has anyone ever tried using this? If so, how was the experience? Thanks in advance. submitted by /u/FlyingRaijinEX [link] [comments]
- One pytest marker to track the performance of your testsby /u/toodarktoshine (Python) on May 2, 2024 at 5:18 am
Hello Pythonistas! I just wrote a blog post about measuring performance inside pytest test cases. We dive into why it’s important to test for performance and how to integrate the measurements in the CI. Here is the link to the blog: https://codspeed.io/blog/one-pytest-marker-to-track-the-performance-of-your-tests submitted by /u/toodarktoshine [link] [comments]
- PkgInspect - Inspect Local/External Python Packagesby /u/yousefabuz (Python) on May 2, 2024 at 2:52 am
GitHub What My Project Does PkgInspect is a comprehensive tool designed to inspect and compare Python packages and Python versions effortlessly. It equips users with a comprehensive set of tools and utility classes to retrieve essential information from installed Python packages, compare versions seamlessly, and extract various details about Python installations with ease. Target Audience Developers and Python enthusiasts looking to streamline the process of inspecting Python packages, comparing versions, and extracting vital information from Python installations will find PkgInspect invaluable. Many current modules such as importlib_metadata and pkg_resources are fairly limited on what items can be inspected and retrieved for a specified python package. Also noticed pkg_resources has also deprecated some of its important retrieval methods. Comparison PkgInspect stands out from other Python package inspection tools due to its robust features. Unlike traditional methods that require manual inspection and comparison, PkgInspect automates the process, saving developers valuable time and effort. With PkgInspect, you can effortlessly retrieve package information, compare versions across different Python installations, and extract crucial details with just a few simple commands. Key Features Inspect Packages: Retrieve comprehensive information about installed Python packages. Compare Versions: Seamlessly compare package data across different Python versions. Retrieve Installed Pythons: Identify and list installed Python versions effortlessly. Inspect PyPI Packages: Gather detailed information about packages from the Python Package Index (PyPI). Fetch Available Updates: Stay up-to-date with available updates for a package from the current version. List Inspection Fieldnames: Access a list of available fieldnames for package inspection. Retrieve Package Metrics: Extract OS statistics about a package effortlessly. Fetch GitHub Statistics: Retrieve insightful statistics about a package from GitHub effortlessly. Retrieve all Python Packages: Easily list all installed Python packages for a given Python version. Main Components Core Modules PkgInspect: Inspects Python packages and retrieves package information. PkgVersions: Retrieves and compares package data across different Python versions. PkgMetrics: Extracts OS statistics about a package. Functions - inspect_package: Inspects a Python package and retrieves package information. - inspect_pypi: Inspects a package from the Python Package Index (PyPI). - get_available_updates: Fetches available updates for a package from the current version. - get_installed_pythons: Identifies and lists installed Python versions. - get_version_packages: Lists all installed Python packages for a given Python version. - pkg_version_compare: Compares package data across different Python versions. Inspection Field Options Any other field name will be treated as a file name to inspect from the packages' site-path directory. - `short_meta` (dict[str, Any]): Returns a dictionary of the most important metadata fields. - If only one field is needed, you can use any of the following metadata fields. - Possible Fields instead of `short_meta`: - `Metadata-Version` (PackageVersion) - `Name` (str) - `Summary` (str) - `Author-email` (str) - `Home-page` (str) - `Download-URL` (str) - `Platform(s)` (set) - `Author` (str) - `Classifier(s)` (set) - `Description-Content-Type` (str) - `short_license` (str): Returns the name of the license being used. - `metadata` (str): Returns the contents of the METADATA file. - `installer` (str): Returns the installer tool used for installation. - `license` (str): Returns the contents of the LICENSE file. - `record` (str): Returns the list of installed files. - `wheel` (str): Returns information about the Wheel distribution format. - `requested` (str): Returns information about the requested installation. - `authors` (str): Returns the contents of the AUTHORS.md file. - `entry_points` (str): Returns the contents of the entry_points.txt file. - `top_level` (str): Returns the contents of the top_level.txt file. - `source_file` (str): Returns the source file path for the specified package. - `source_code` (str): Returns the source code contents for the specified package. - `doc` (str): Returns the documentation for the specified package. - `Pkg` Custom Class Fields - `PkgInspect fields`: Possible Fields from the `PkgInspect` class. - `site_path` (Path): Returns the site path of the package. - `package_paths` (Iterable[Path]): Returns the package paths of the package. - `package_versions` (Generator[tuple[str, tuple[tuple[Any, str]]]]): Returns the package versions of the package. - `pyversions` (tuple[Path]): Returns the Python versions of the package. - `installed_pythons` (TupleOfPkgVersions): Returns the installed Python versions of the package. - `site_packages` (Iterable[str]): Returns the site packages of the package. - `islatest_version` (bool): Returns True if the package is the latest version. - `isinstalled_version` (bool): Returns True if the package is the installed version. - `installed_version` (PackageVersion): Returns the installed version of the package. - `available_updates` (TupleOfPkgVersions): Returns the available updates of the package. - `PkgVersions fields`: Possible Fields from the `PkgVersions` class. - `initial_version` (PackageVersion): Returns the initial version of the package. - `installed_version` (PackageVersion): Returns the installed version of the package. - `latest_version` (PackageVersion): Returns the latest version of the package. - `total_versions` (int): Returns the total number of versions of the package. - `version_history` (TupleOfPkgVersions): Returns the version history of the specified package. - `package_url`: Returns the URL of the package on PyPI. - `github_stats_url` (str): Returns the GitHub statistics URL of the package. - `github_stats` (dict[str, Any]): Returns the GitHub statistics of the package. - The GitHub statistics are returned as a dictionary \ containing the following fields which can accessed using the `item` parameter: - `Forks` (int): Returns the number of forks on GitHub. - `Stars` (int): Returns the number of stars on GitHub. - `Watchers` (int): Returns the number of watchers on GitHub. - `Contributors` (int): Returns the number of contributors on GitHub. - `Dependencies` (int): Returns the number of dependencies on GitHub. - `Dependent repositories` (int): Returns the number of dependent repositories on GitHub. - `Dependent packages` (int): Returns the number of dependent packages on GitHub. - `Repository size` (NamedTuple): Returns the size of the repository on GitHub. - `SourceRank` (int): Returns the SourceRank of the package on GitHub. - `Total releases` (int): Returns the total number of releases on GitHub. - `PkgMetrics fields`: Possible Fields from the `PkgMetrics` class. - `all_metric_stats` (dict[str, Any]): Returns all the OS statistics of the package. - `total_size` (int): Returns the total size of the package. - `date_installed` (datetime): Returns the date the package was installed. - `pypistats fields`: Possible Fields from the `pypistats` module. - `all-pypi-stats` (dict[str, Any]): Returns all the statistics of the package on PyPI into a single dictionary. - `stats-overall` (dict[str, Any]): Returns the overall statistics of the package on PyPI. - `stats-major` (dict[str, Any]): Returns the major version statistics of the package on PyPI. - `stats-minor` (dict[str, Any]): Returns the minor version statistics of the package on PyPI. - `stats-recent` (dict[str, Any]): Returns the recent statistics of the package on PyPI. - `stats-system` (dict[str, Any]): Returns the system statistics of the package on PyPI. Downsides & Limitations My algorithms are fairly well but do come with some important downsides. PkgInspect will ONLY inspect packages that are python files or contains a dist-info folder in the site-packages folder for a given Python version. Was not able to efficiently figure out a way to retrieve all necessary packages without containing unrelevant folders/files. Some personal packages may be skipped otherwise. Beta (pre-releases) has not been implemented yet. As many files may be handled, the runtime may be slow for some users. The demand for a project like this is not so much in-demand but have noticed many people, including my self, still seeking for a project like this. However, this type of project does seem to exceed my experience level with Python and algorithms (hence the downsides) so not entirely sure how far this project may come in the future. Was hoping for it to be GUI based if possible. Usage Examples from pkg_inspect import inspect_package inspect_package("pkg_inspect", itemOrfile="initial_version") # Output (Format - DateTimeAndVersion): ('May 02, 2024', '0.1.0') inspect_package("pkg_inspect", itemOrfile="version_history") # Output (Format - tuple[DateTimeAndVersion]): (('May 02, 2024', '0.1.2'), ('May 02, 2024', '0.1.1'), ('May 02, 2024', '0.1.0')) inspect_package("pkg_inspect", pyversion="3.12", itemOrfile="short_meta") # Output (Format dict[str, Any]): {'Author': 'Yousef Abuzahrieh', 'Author-email': 'yousefzahrieh17@gmail.com', 'Classifiers': {'Development Status 4 Beta', 'Intended Audience Developers', 'License OSI Approved Apache Software License', 'Operating System OS Independent', 'Programming Language Python 3', 'Programming Language Python 3 Only', 'Topic Utilities'}, 'Description-Content-Type': 'text/markdown', 'Download-URL': 'https://github.com/yousefabuz17/PkgInspect.git', 'Home-page': 'https://github.com/yousefabuz17/PkgInspect', 'License': 'Apache Software License', 'Metadata-Version': <Version('2.1')>, 'Name': 'pkg-inspect', 'Platforms': {'Windows', 'MacOS', 'Linux'}, 'Summary': 'A comprehensive tools to inspect Python packages and Python ' 'installations.'} inspect_package("pandas", pyversion="3.12", itemOrfile="github_stats") # Output (Format - dict[str, Any]): {'Contributors': '1.09K', 'Dependencies': 3, 'Dependent packages': '41.3K', 'Dependent repositories': '38.4K', 'Forks': '17.3K', 'Repository size': Stats(symbolic='338.000 KB (Kilobytes)', calculated_size=338.0, bytes_size=346112.0), 'SourceRank': 32, 'Stars': '41.9K', 'Total releases': 126, 'Watchers': 1116} submitted by /u/yousefabuz [link] [comments]
- How to create architecture diagrams from code in Jupyter Notebookby /u/writer_on_rails (Python) on May 2, 2024 at 2:32 am
Hello world,I wrote an article about creating diagrams from code on Jupyter Notebook inside VS Code. It will give you a brief on the setup and also an overview of concepts. Within 5 minutes, you should be able to start making cool architecture diagrams. [TO MODERATOR: This link does not contain any paywalled or paid content. All the contents are available for free] Article link: https://ashgaikwad.substack.com/p/how-to-create-architecture-diagrams submitted by /u/writer_on_rails [link] [comments]
- Thursday Daily Thread: Python Careers, Courses, and Furthering Education!by /u/AutoModerator (Python) on May 2, 2024 at 12:00 am
Weekly Thread: Professional Use, Jobs, and Education 🏢 Welcome to this week's discussion on Python in the professional world! This is your spot to talk about job hunting, career growth, and educational resources in Python. Please note, this thread is not for recruitment. How it Works: Career Talk: Discuss using Python in your job, or the job market for Python roles. Education Q&A: Ask or answer questions about Python courses, certifications, and educational resources. Workplace Chat: Share your experiences, challenges, or success stories about using Python professionally. Guidelines: This thread is not for recruitment. For job postings, please see r/PythonJobs or the recruitment thread in the sidebar. Keep discussions relevant to Python in the professional and educational context. Example Topics: Career Paths: What kinds of roles are out there for Python developers? Certifications: Are Python certifications worth it? Course Recommendations: Any good advanced Python courses to recommend? Workplace Tools: What Python libraries are indispensable in your professional work? Interview Tips: What types of Python questions are commonly asked in interviews? Let's help each other grow in our careers and education. Happy discussing! 🌟 submitted by /u/AutoModerator [link] [comments]
Active Hydrating Toner, Anti-Aging Replenishing Advanced Face Moisturizer, with Vitamins A, C, E & Natural Botanicals to Promote Skin Balance & Collagen Production, 6.7 Fl Oz
Age Defying 0.3% Retinol Serum, Anti-Aging Dark Spot Remover for Face, Fine Lines & Wrinkle Pore Minimizer, with Vitamin E & Natural Botanicals
Firming Moisturizer, Advanced Hydrating Facial Replenishing Cream, with Hyaluronic Acid, Resveratrol & Natural Botanicals to Restore Skin's Strength, Radiance, and Resilience, 1.75 Oz
Skin Stem Cell Serum
Smartphone 101 - Pick a smartphone for me - android or iOS - Apple iPhone or Samsung Galaxy or Huawei or Xaomi or Google Pixel
Can AI Really Predict Lottery Results? We Asked an Expert.
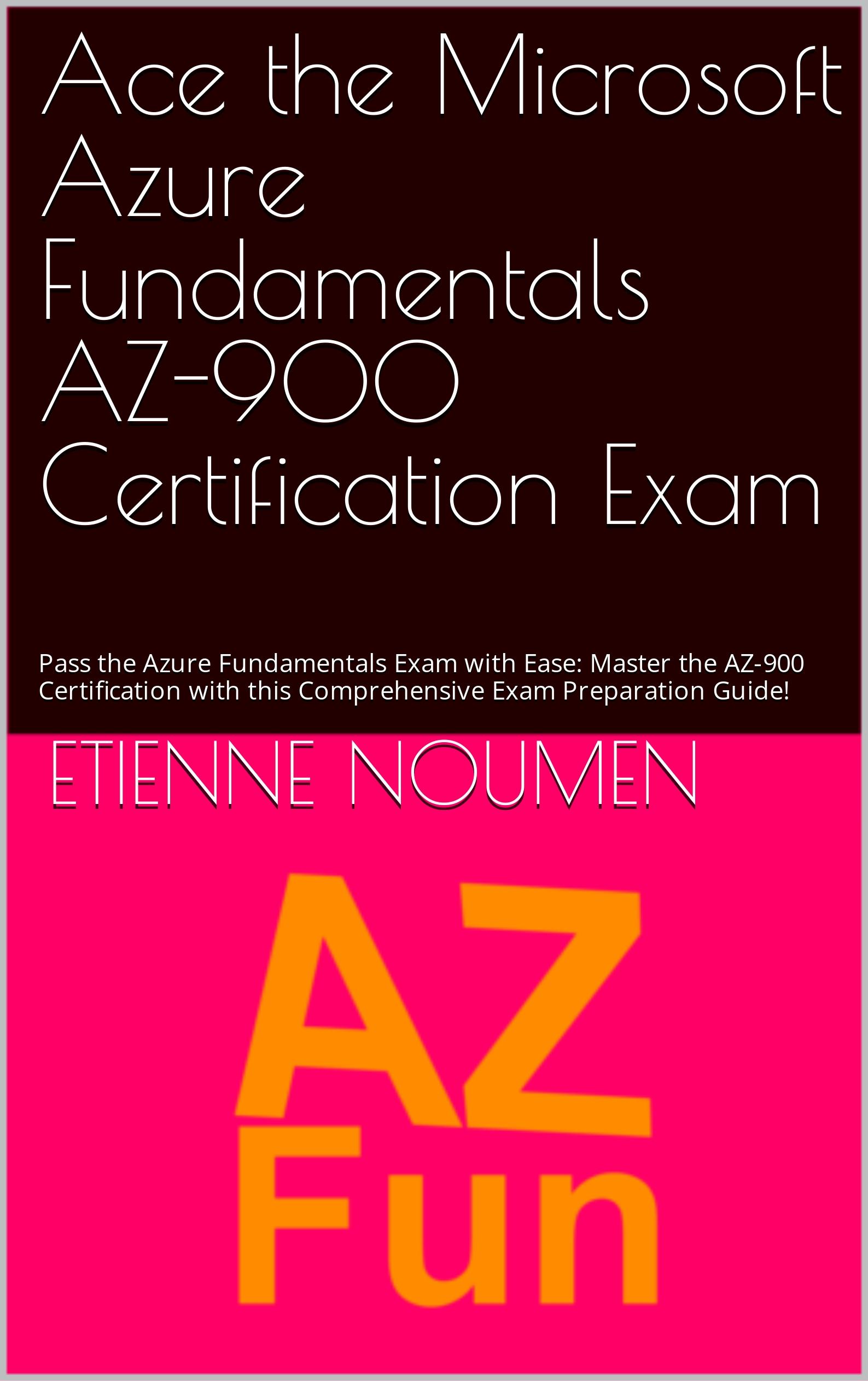

Djamgatech

Read Photos and PDFs Aloud for me iOS
Read Photos and PDFs Aloud for me android
Read Photos and PDFs Aloud For me Windows 10/11
Read Photos and PDFs Aloud For Amazon
Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE (Email us for more)
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes: 96DRHDRA9J7GTN6(Email us for more)
FREE 10000+ Quiz Trivia and and Brain Teasers for All Topics including Cloud Computing, General Knowledge, History, Television, Music, Art, Science, Movies, Films, US History, Soccer Football, World Cup, Data Science, Machine Learning, Geography, etc....

List of Freely available programming books - What is the single most influential book every Programmers should read
- Bjarne Stroustrup - The C++ Programming Language
- Brian W. Kernighan, Rob Pike - The Practice of Programming
- Donald Knuth - The Art of Computer Programming
- Ellen Ullman - Close to the Machine
- Ellis Horowitz - Fundamentals of Computer Algorithms
- Eric Raymond - The Art of Unix Programming
- Gerald M. Weinberg - The Psychology of Computer Programming
- James Gosling - The Java Programming Language
- Joel Spolsky - The Best Software Writing I
- Keith Curtis - After the Software Wars
- Richard M. Stallman - Free Software, Free Society
- Richard P. Gabriel - Patterns of Software
- Richard P. Gabriel - Innovation Happens Elsewhere
- Code Complete (2nd edition) by Steve McConnell
- The Pragmatic Programmer
- Structure and Interpretation of Computer Programs
- The C Programming Language by Kernighan and Ritchie
- Introduction to Algorithms by Cormen, Leiserson, Rivest & Stein
- Design Patterns by the Gang of Four
- Refactoring: Improving the Design of Existing Code
- The Mythical Man Month
- The Art of Computer Programming by Donald Knuth
- Compilers: Principles, Techniques and Tools by Alfred V. Aho, Ravi Sethi and Jeffrey D. Ullman
- Gödel, Escher, Bach by Douglas Hofstadter
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
- Effective C++
- More Effective C++
- CODE by Charles Petzold
- Programming Pearls by Jon Bentley
- Working Effectively with Legacy Code by Michael C. Feathers
- Peopleware by Demarco and Lister
- Coders at Work by Peter Seibel
- Surely You're Joking, Mr. Feynman!
- Effective Java 2nd edition
- Patterns of Enterprise Application Architecture by Martin Fowler
- The Little Schemer
- The Seasoned Schemer
- Why's (Poignant) Guide to Ruby
- The Inmates Are Running The Asylum: Why High Tech Products Drive Us Crazy and How to Restore the Sanity
- The Art of Unix Programming
- Test-Driven Development: By Example by Kent Beck
- Practices of an Agile Developer
- Don't Make Me Think
- Agile Software Development, Principles, Patterns, and Practices by Robert C. Martin
- Domain Driven Designs by Eric Evans
- The Design of Everyday Things by Donald Norman
- Modern C++ Design by Andrei Alexandrescu
- Best Software Writing I by Joel Spolsky
- The Practice of Programming by Kernighan and Pike
- Pragmatic Thinking and Learning: Refactor Your Wetware by Andy Hunt
- Software Estimation: Demystifying the Black Art by Steve McConnel
- The Passionate Programmer (My Job Went To India) by Chad Fowler
- Hackers: Heroes of the Computer Revolution
- Algorithms + Data Structures = Programs
- Writing Solid Code
- JavaScript - The Good Parts
- Getting Real by 37 Signals
- Foundations of Programming by Karl Seguin
- Computer Graphics: Principles and Practice in C (2nd Edition)
- Thinking in Java by Bruce Eckel
- The Elements of Computing Systems
- Refactoring to Patterns by Joshua Kerievsky
- Modern Operating Systems by Andrew S. Tanenbaum
- The Annotated Turing
- Things That Make Us Smart by Donald Norman
- The Timeless Way of Building by Christopher Alexander
- The Deadline: A Novel About Project Management by Tom DeMarco
- The C++ Programming Language (3rd edition) by Stroustrup
- Patterns of Enterprise Application Architecture
- Computer Systems - A Programmer's Perspective
- Agile Principles, Patterns, and Practices in C# by Robert C. Martin
- Growing Object-Oriented Software, Guided by Tests
- Framework Design Guidelines by Brad Abrams
- Object Thinking by Dr. David West
- Advanced Programming in the UNIX Environment by W. Richard Stevens
- Hackers and Painters: Big Ideas from the Computer Age
- The Soul of a New Machine by Tracy Kidder
- CLR via C# by Jeffrey Richter
- The Timeless Way of Building by Christopher Alexander
- Design Patterns in C# by Steve Metsker
- Alice in Wonderland by Lewis Carol
- Zen and the Art of Motorcycle Maintenance by Robert M. Pirsig
- About Face - The Essentials of Interaction Design
- Here Comes Everybody: The Power of Organizing Without Organizations by Clay Shirky
- The Tao of Programming
- Computational Beauty of Nature
- Writing Solid Code by Steve Maguire
- Philip and Alex's Guide to Web Publishing
- Object-Oriented Analysis and Design with Applications by Grady Booch
- Effective Java by Joshua Bloch
- Computability by N. J. Cutland
- Masterminds of Programming
- The Tao Te Ching
- The Productive Programmer
- The Art of Deception by Kevin Mitnick
- The Career Programmer: Guerilla Tactics for an Imperfect World by Christopher Duncan
- Paradigms of Artificial Intelligence Programming: Case studies in Common Lisp
- Masters of Doom
- Pragmatic Unit Testing in C# with NUnit by Andy Hunt and Dave Thomas with Matt Hargett
- How To Solve It by George Polya
- The Alchemist by Paulo Coelho
- Smalltalk-80: The Language and its Implementation
- Writing Secure Code (2nd Edition) by Michael Howard
- Introduction to Functional Programming by Philip Wadler and Richard Bird
- No Bugs! by David Thielen
- Rework by Jason Freid and DHH
- JUnit in Action
#BlackOwned #BlackEntrepreneurs #BlackBuniness #AWSCertified #AWSCloudPractitioner #AWSCertification #AWSCLFC02 #CloudComputing #AWSStudyGuide #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AWSBasics #AWSCertified #AWSMachineLearning #AWSCertification #AWSSpecialty #MachineLearning #AWSStudyGuide #CloudComputing #DataScience #AWSCertified #AWSSolutionsArchitect #AWSArchitectAssociate #AWSCertification #AWSStudyGuide #CloudComputing #AWSArchitecture #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AzureFundamentals #AZ900 #MicrosoftAzure #ITCertification #CertificationPrep #StudyMaterials #TechLearning #MicrosoftCertified #AzureCertification #TechBooks
Top 1000 Canada Quiz and trivia: CANADA CITIZENSHIP TEST- HISTORY - GEOGRAPHY - GOVERNMENT- CULTURE - PEOPLE - LANGUAGES - TRAVEL - WILDLIFE - HOCKEY - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION

Top 1000 Africa Quiz and trivia: HISTORY - GEOGRAPHY - WILDLIFE - CULTURE - PEOPLE - LANGUAGES - TRAVEL - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION

Exploring the Pros and Cons of Visiting All Provinces and Territories in Canada.

Exploring the Advantages and Disadvantages of Visiting All 50 States in the USA

Health Health, a science-based community to discuss health news and the coronavirus (COVID-19) pandemic
- Found: the dial in the brain that controls the immune systemby /u/barweis on May 3, 2024 at 3:18 pm
submitted by /u/barweis [link] [comments]
- Long Beach health officials declare tuberculosis outbreak a public health emergencyby /u/josh252 on May 3, 2024 at 11:26 am
submitted by /u/josh252 [link] [comments]
- Why does TB have such a hold on the Inuit communities of the Canadian Arctic?by /u/Maxcactus on May 3, 2024 at 10:11 am
submitted by /u/Maxcactus [link] [comments]
- Launching an effective bird flu vaccine quickly could be tough, scientists warnby /u/Maxcactus on May 3, 2024 at 10:01 am
submitted by /u/Maxcactus [link] [comments]
- What are the most frequent and serious causes of child poisoning?by /u/euronews-english on May 3, 2024 at 9:43 am
submitted by /u/euronews-english [link] [comments]
Today I Learned (TIL) You learn something new every day; what did you learn today? Submit interesting and specific facts about something that you just found out here.
- TIL John Walsh, host of "America's Most Wanted," became an advocate for missing children after his son Adam was abducted and murdered in 1981. His advocacy led to changes in laws and the creation of the National Center for Missing & Exploited Children. His show helped capture over 1,200 fugitives.by /u/whstlngisnvrenf on May 3, 2024 at 2:00 pm
submitted by /u/whstlngisnvrenf [link] [comments]
- TIL - Computers were people (mostly women) up until WWII. Teams of people, often women from the late nineteenth century onwards, were used to undertake long and often tedious calculations.by /u/MyHamburgerLovesMe on May 3, 2024 at 1:44 pm
submitted by /u/MyHamburgerLovesMe [link] [comments]
- TIL when a peanut plant is pollinated, the flower loses its petals. The bare flower bud hangs from the stem and grows down toward the ground until it penetrates the soil. Once it reaches the appropriate depth, it becomes a peanutby /u/admiralturtleship on May 3, 2024 at 1:38 pm
submitted by /u/admiralturtleship [link] [comments]
- TIL that 3% of people in the US will have a psychotic break at some point in their livesby /u/Just_Want_To_Write on May 3, 2024 at 1:08 pm
submitted by /u/Just_Want_To_Write [link] [comments]
- TIL Most of the stories about the Dvorak keyboard being superior to the standard QWERTY come from a Navy study conducted by August Dvorak, who owned the patent on the Dvorak keyoard.by /u/littletoyboat on May 3, 2024 at 11:00 am
submitted by /u/littletoyboat [link] [comments]
Reddit Science This community is a place to share and discuss new scientific research. Read about the latest advances in astronomy, biology, medicine, physics, social science, and more. Find and submit new publications and popular science coverage of current research.
- Researchers identify 2 monoclonal antibodies against Nipah virus through phage display. As there are no immunotherapies against this virus, these findings could assist in the development of antiviral drugs and vaccine design.by /u/Biointron on May 3, 2024 at 3:43 pm
submitted by /u/Biointron [link] [comments]
- Texas dairy farm worker's case may be first where H5N1 bird flu virus spread from mammal to human according to a new report by the CDC. All previous human cases were linked to transmission from infected birds.by /u/shiruken on May 3, 2024 at 3:11 pm
submitted by /u/shiruken [link] [comments]
- The environmental sustainability of digital content consumptionby /u/mvdm_42 on May 3, 2024 at 2:56 pm
submitted by /u/mvdm_42 [link] [comments]
- Transparent filter with 10,000-pixel power turns phone into pro camera | The study utilizes a transparent, one-centimeter-square plane featuring a 2D semiconductor—a thin film composed of only a few atoms.by /u/chrisdh79 on May 3, 2024 at 2:31 pm
submitted by /u/chrisdh79 [link] [comments]
- Only half of studies in 5 leading journals of animal behavior research report using techniques to avoid observer bias from sneaking in – but that’s up from under 20% in study 8 years earlierby /u/globehater on May 3, 2024 at 1:08 pm
submitted by /u/globehater [link] [comments]
Reddit Sports Sports News and Highlights from the NFL, NBA, NHL, MLB, MLS, and leagues around the world.
- Chevrolet denies participation in Team Penske's IndyCar cheating scandalby /u/Oldtimer_2 on May 3, 2024 at 3:49 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Larry Demeritte is just the second Black trainer since 1951 to saddle a horse for the Kentucky Derbyby /u/Oldtimer_2 on May 3, 2024 at 3:48 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Buffalo Bills sign WR Chase Claypool to a one year contractby /u/Oldtimer_2 on May 3, 2024 at 3:43 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Report: Beckham to sign 1-year deal with Dolphinsby /u/SpiritedSuccess5675 on May 3, 2024 at 3:30 pm
submitted by /u/SpiritedSuccess5675 [link] [comments]
- Verstappen says future is with Red Bull, suggests €250M not enough to switch. [$275M US]by /u/Oldtimer_2 on May 3, 2024 at 3:20 pm
submitted by /u/Oldtimer_2 [link] [comments]