As a web developer, can you explain why React is needed?
In the early days of the internet, web sites were essentially made of static HTML files. Web servers were little more than file servers, when a user would come to a url, the web server would simply fetch it, and send it to the user via their browser, along with all kind of assets, like fonts and images.

The functionality of this kind of web pages are very limited, so eventually the web became more dynamic. When people would visit a page or interact with a form, instead of just fetching data, the server could perform an operation and prepare some content on demand. That content would still be sent to the user’s browser. There could also a little bit of code running on the browser, to animate pages, handle form and what not, but not very much.
So up until around 2010, that was the dominant model. Code could be involved to generate content but the browser wouldn’t do much, most of the logic would happen on servers which would just send prepared content to the browser.
However, in the early 2010s, this paradigm started to shift. With HTML5/CSS3, the browser became much more capable, and so people started to move the logic that would generate content from the server to the browser. Instead of sending a whole styled HTML page, a web server could just send the data needed to create it. Then, code could run on the browser to actually turn that data into HTML. That browser code could also update what the user would see, making just the required data calls.
So, in the early to mid 2010s, front-end code would typically:
- render complex web pages from data retrieved from back-end,
- simulate “navigation” between different views: when the user would do some actions, the entire page would change, the url would update etc. but without actually loading a new page from the server.
- maintain the state of an application: the application could track certain things about the user and the session, and won’t have to reload that information from the server all the time.
- dynamically update both contents and style of a web page.
Now, all of this is possible to do in “vanilla javascript”. But it’s really cumbersome to implement it, and especially tricky to do it in a performant way. There are millions and millions of “web apps” that are replacing the static “web sites” of old, and which all need to dynamically render content. Should developers reimplement that from scratch each time?
Enter the web frameworks such as React. These frameworks are abstractions that let the developers focus on the logic of their web app (where the data comes from, how content is organized) without being tied to the nitty gritty. Web frameworks make developers organize their code in building blocks called modules or components. Somebody could write a header component and someone else building a page could reuse that header component. And a third developer could change the header component, and that change would be reflected everywhere the component is used. Folks could also build 3rd party libraries compatible with the web framework ecosystem, that would address common problems that many developers face. For instance, someone could create a date picker component (a notably tricky interface) that anyone can reuse and customize. Or create a solution to deal with very long pages by only rendering what is in the browser viewport, and creating/deleting elements as a user would scroll.
To have the support of this ecosystem is a huge productivity boost. There are millions of developers who work with React, and the most popular React libraries are very elegant solutions to hard problems(the same could be said of Angular, Vue etc. though their communities are a bit smaller).
React and web frameworks aren’t exactly needed, in fact there is a reverse trend in the last couple of years to go back to server generated content in some cases or to only use vanilla javascript, but it’s a very solid foundation to build a web app.

Comments:
1- The specific rationale for React is state management and efficient page updates, it’s underlying power comes not just from the structure and tooling provided by it being a framework, but also the shadow-DOM and component lifecycle that along with state management empower greater interactivity without very slow inefficient page updates.
2- React isn’t needed, but it is a great framework that can reduce the amount of work you do in making a website/webapp.
React is great for widgets and implementing patterns. You can keep data/text separate from structure and behavior. React, angular and vue are all popular frameworks. Before that we used stuff like dust, handlebars, jQuery and UI libraries like dojo and jQuery UI.
Developers are always looking for ways to be more efficient and more maintainable. React is a current iteration tool for being more efficient.
3- It is needed as a pattern for the devs to create packages that will works (The React packages). In NPM there are many packages, but all them are following its own logic, docs or no docs, they are based on another packages, etc. With things like React, you are somehow limited to follow its rules and you are entering its ecosystem which is good. This is true for all frameworks/libraries.
Advertise with us - Post Your Good Content Here
We are ranked in the Top 20 on Google
AI Dashboard is available on the Web, Apple, Google, and Microsoft, PRO version
React also has some configurations which follows the best practices (create-react-app, NextJS, etc), but this is the same and for others.
The difference is that React is close to JS and there is a lot of freedom, what to use like a package, starter pack, use or not Typescript.
Active Hydrating Toner, Anti-Aging Replenishing Advanced Face Moisturizer, with Vitamins A, C, E & Natural Botanicals to Promote Skin Balance & Collagen Production, 6.7 Fl Oz
Age Defying 0.3% Retinol Serum, Anti-Aging Dark Spot Remover for Face, Fine Lines & Wrinkle Pore Minimizer, with Vitamin E & Natural Botanicals
Firming Moisturizer, Advanced Hydrating Facial Replenishing Cream, with Hyaluronic Acid, Resveratrol & Natural Botanicals to Restore Skin's Strength, Radiance, and Resilience, 1.75 Oz
Skin Stem Cell Serum
Smartphone 101 - Pick a smartphone for me - android or iOS - Apple iPhone or Samsung Galaxy or Huawei or Xaomi or Google Pixel
Can AI Really Predict Lottery Results? We Asked an Expert.
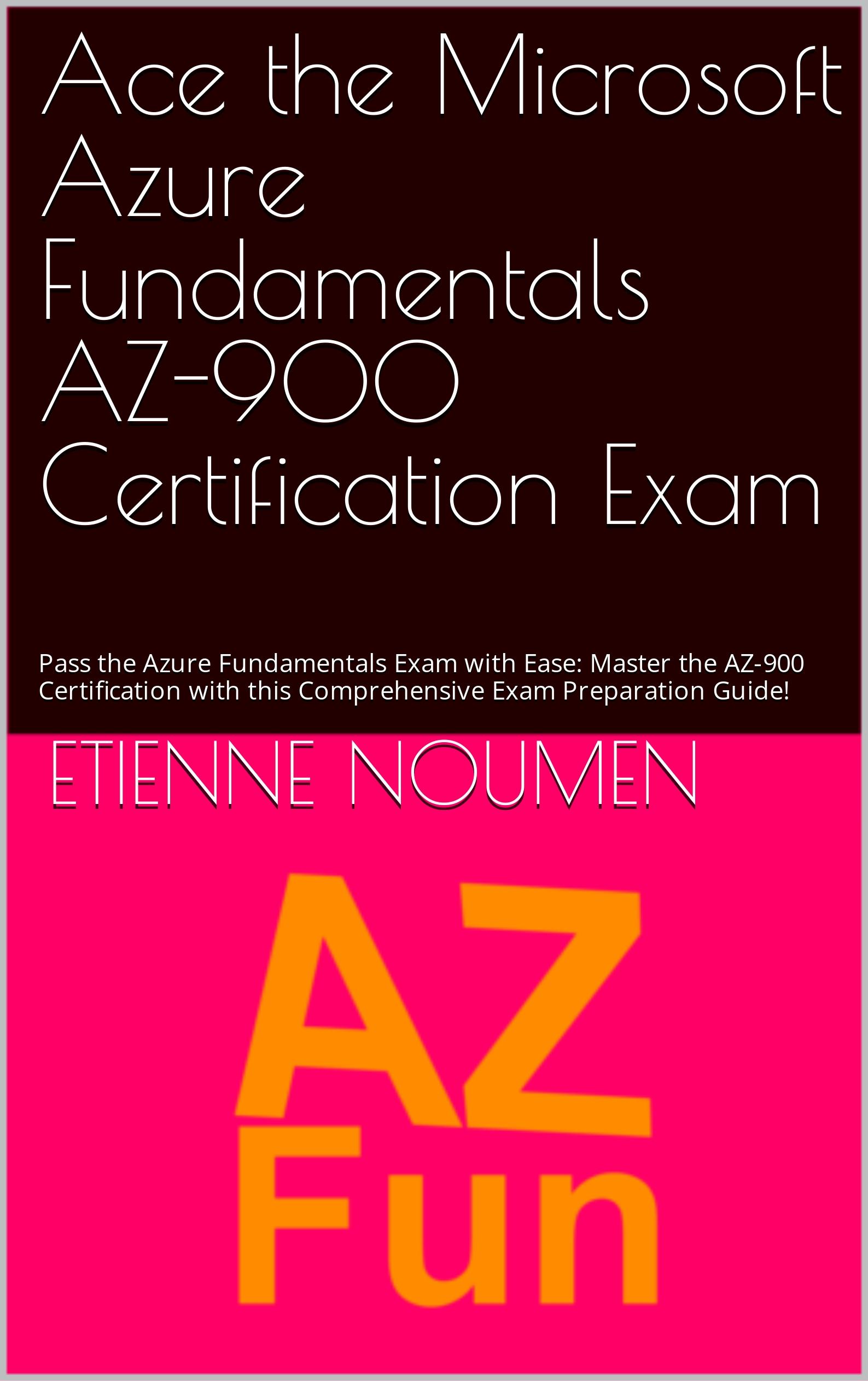

Djamgatech

Read Photos and PDFs Aloud for me iOS
Read Photos and PDFs Aloud for me android
Read Photos and PDFs Aloud For me Windows 10/11
Read Photos and PDFs Aloud For Amazon
Get 20% off Google Workspace (Google Meet) Business Plan (AMERICAS): M9HNXHX3WC9H7YE (Email us for more)
Get 20% off Google Google Workspace (Google Meet) Standard Plan with the following codes: 96DRHDRA9J7GTN6(Email us for more)
FREE 10000+ Quiz Trivia and and Brain Teasers for All Topics including Cloud Computing, General Knowledge, History, Television, Music, Art, Science, Movies, Films, US History, Soccer Football, World Cup, Data Science, Machine Learning, Geography, etc....

List of Freely available programming books - What is the single most influential book every Programmers should read
- Bjarne Stroustrup - The C++ Programming Language
- Brian W. Kernighan, Rob Pike - The Practice of Programming
- Donald Knuth - The Art of Computer Programming
- Ellen Ullman - Close to the Machine
- Ellis Horowitz - Fundamentals of Computer Algorithms
- Eric Raymond - The Art of Unix Programming
- Gerald M. Weinberg - The Psychology of Computer Programming
- James Gosling - The Java Programming Language
- Joel Spolsky - The Best Software Writing I
- Keith Curtis - After the Software Wars
- Richard M. Stallman - Free Software, Free Society
- Richard P. Gabriel - Patterns of Software
- Richard P. Gabriel - Innovation Happens Elsewhere
- Code Complete (2nd edition) by Steve McConnell
- The Pragmatic Programmer
- Structure and Interpretation of Computer Programs
- The C Programming Language by Kernighan and Ritchie
- Introduction to Algorithms by Cormen, Leiserson, Rivest & Stein
- Design Patterns by the Gang of Four
- Refactoring: Improving the Design of Existing Code
- The Mythical Man Month
- The Art of Computer Programming by Donald Knuth
- Compilers: Principles, Techniques and Tools by Alfred V. Aho, Ravi Sethi and Jeffrey D. Ullman
- Gödel, Escher, Bach by Douglas Hofstadter
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
- Effective C++
- More Effective C++
- CODE by Charles Petzold
- Programming Pearls by Jon Bentley
- Working Effectively with Legacy Code by Michael C. Feathers
- Peopleware by Demarco and Lister
- Coders at Work by Peter Seibel
- Surely You're Joking, Mr. Feynman!
- Effective Java 2nd edition
- Patterns of Enterprise Application Architecture by Martin Fowler
- The Little Schemer
- The Seasoned Schemer
- Why's (Poignant) Guide to Ruby
- The Inmates Are Running The Asylum: Why High Tech Products Drive Us Crazy and How to Restore the Sanity
- The Art of Unix Programming
- Test-Driven Development: By Example by Kent Beck
- Practices of an Agile Developer
- Don't Make Me Think
- Agile Software Development, Principles, Patterns, and Practices by Robert C. Martin
- Domain Driven Designs by Eric Evans
- The Design of Everyday Things by Donald Norman
- Modern C++ Design by Andrei Alexandrescu
- Best Software Writing I by Joel Spolsky
- The Practice of Programming by Kernighan and Pike
- Pragmatic Thinking and Learning: Refactor Your Wetware by Andy Hunt
- Software Estimation: Demystifying the Black Art by Steve McConnel
- The Passionate Programmer (My Job Went To India) by Chad Fowler
- Hackers: Heroes of the Computer Revolution
- Algorithms + Data Structures = Programs
- Writing Solid Code
- JavaScript - The Good Parts
- Getting Real by 37 Signals
- Foundations of Programming by Karl Seguin
- Computer Graphics: Principles and Practice in C (2nd Edition)
- Thinking in Java by Bruce Eckel
- The Elements of Computing Systems
- Refactoring to Patterns by Joshua Kerievsky
- Modern Operating Systems by Andrew S. Tanenbaum
- The Annotated Turing
- Things That Make Us Smart by Donald Norman
- The Timeless Way of Building by Christopher Alexander
- The Deadline: A Novel About Project Management by Tom DeMarco
- The C++ Programming Language (3rd edition) by Stroustrup
- Patterns of Enterprise Application Architecture
- Computer Systems - A Programmer's Perspective
- Agile Principles, Patterns, and Practices in C# by Robert C. Martin
- Growing Object-Oriented Software, Guided by Tests
- Framework Design Guidelines by Brad Abrams
- Object Thinking by Dr. David West
- Advanced Programming in the UNIX Environment by W. Richard Stevens
- Hackers and Painters: Big Ideas from the Computer Age
- The Soul of a New Machine by Tracy Kidder
- CLR via C# by Jeffrey Richter
- The Timeless Way of Building by Christopher Alexander
- Design Patterns in C# by Steve Metsker
- Alice in Wonderland by Lewis Carol
- Zen and the Art of Motorcycle Maintenance by Robert M. Pirsig
- About Face - The Essentials of Interaction Design
- Here Comes Everybody: The Power of Organizing Without Organizations by Clay Shirky
- The Tao of Programming
- Computational Beauty of Nature
- Writing Solid Code by Steve Maguire
- Philip and Alex's Guide to Web Publishing
- Object-Oriented Analysis and Design with Applications by Grady Booch
- Effective Java by Joshua Bloch
- Computability by N. J. Cutland
- Masterminds of Programming
- The Tao Te Ching
- The Productive Programmer
- The Art of Deception by Kevin Mitnick
- The Career Programmer: Guerilla Tactics for an Imperfect World by Christopher Duncan
- Paradigms of Artificial Intelligence Programming: Case studies in Common Lisp
- Masters of Doom
- Pragmatic Unit Testing in C# with NUnit by Andy Hunt and Dave Thomas with Matt Hargett
- How To Solve It by George Polya
- The Alchemist by Paulo Coelho
- Smalltalk-80: The Language and its Implementation
- Writing Secure Code (2nd Edition) by Michael Howard
- Introduction to Functional Programming by Philip Wadler and Richard Bird
- No Bugs! by David Thielen
- Rework by Jason Freid and DHH
- JUnit in Action
#BlackOwned #BlackEntrepreneurs #BlackBuniness #AWSCertified #AWSCloudPractitioner #AWSCertification #AWSCLFC02 #CloudComputing #AWSStudyGuide #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AWSBasics #AWSCertified #AWSMachineLearning #AWSCertification #AWSSpecialty #MachineLearning #AWSStudyGuide #CloudComputing #DataScience #AWSCertified #AWSSolutionsArchitect #AWSArchitectAssociate #AWSCertification #AWSStudyGuide #CloudComputing #AWSArchitecture #AWSTraining #AWSCareer #AWSExamPrep #AWSCommunity #AWSEducation #AzureFundamentals #AZ900 #MicrosoftAzure #ITCertification #CertificationPrep #StudyMaterials #TechLearning #MicrosoftCertified #AzureCertification #TechBooks
Top 1000 Canada Quiz and trivia: CANADA CITIZENSHIP TEST- HISTORY - GEOGRAPHY - GOVERNMENT- CULTURE - PEOPLE - LANGUAGES - TRAVEL - WILDLIFE - HOCKEY - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION

Top 1000 Africa Quiz and trivia: HISTORY - GEOGRAPHY - WILDLIFE - CULTURE - PEOPLE - LANGUAGES - TRAVEL - TOURISM - SCENERIES - ARTS - DATA VISUALIZATION

Exploring the Pros and Cons of Visiting All Provinces and Territories in Canada.

Exploring the Advantages and Disadvantages of Visiting All 50 States in the USA

Health Health, a science-based community to discuss health news and the coronavirus (COVID-19) pandemic
- The pull-out method: Why this common contraceptive fails to deliverby /u/Kampala_Dispatch on July 26, 2024 at 7:51 pm
submitted by /u/Kampala_Dispatch [link] [comments]
- Health Canada data reveals surprising number of adverse cannabis reactions (spoiler: it's small)by /u/carajuana_readit on July 26, 2024 at 5:49 pm
submitted by /u/carajuana_readit [link] [comments]
- Online portals deliver scary health news before doctors can weigh inby /u/washingtonpost on July 26, 2024 at 4:37 pm
submitted by /u/washingtonpost [link] [comments]
- Vaccine 'sharply cuts risk of dementia' new study findsby /u/SubstantialSnow7114 on July 26, 2024 at 1:53 pm
submitted by /u/SubstantialSnow7114 [link] [comments]
- Calls to limit sexual partners as mpox makes a resurgence in Australiaby /u/boppinmule on July 26, 2024 at 12:31 pm
submitted by /u/boppinmule [link] [comments]
Today I Learned (TIL) You learn something new every day; what did you learn today? Submit interesting and specific facts about something that you just found out here.
- TIL that in Thailand, if your spouse cheats on you, you can legally sue their lover for damages and can receive up to 5,000,000 THB ($140,000 USD) or more under Section 1523 of the Thai Civil and Commercial Codeby /u/Mavrokordato on July 26, 2024 at 6:57 pm
submitted by /u/Mavrokordato [link] [comments]
- TIL that with a population of 170 million people, Bangladesh is the most populous country to have never won a medal at the Olympic Games.by /u/Blackraven2007 on July 26, 2024 at 6:49 pm
submitted by /u/Blackraven2007 [link] [comments]
- TIL a psychologist got himself admitted to a mental hospital by claiming he heard the words "empty", "hollow" and "thud" in his head. Then, it took him two months to convince them he was sane, after agreeing he was insane and accepting medication.by /u/Hadeverse-050 on July 26, 2024 at 6:44 pm
submitted by /u/Hadeverse-050 [link] [comments]
- TIL Senator John Edwards of NC, USA cheated on his wife and had a child with another woman. He tried to deny it but eventually caved and admitted his mistake. He used campaign funds and was indicted by a grand jury. His life story inspired the show "The Good Wife" by Robert & Michelle Kingby /u/AdvisorPast637 on July 26, 2024 at 6:09 pm
submitted by /u/AdvisorPast637 [link] [comments]
- TIL Zhang Shuhong was a Chinese businessman who committed suicide after toys made at his factory for Fisher-Price (a division of Mattel) were found to contain lead paintby /u/Hopeful-Candle-4884 on July 26, 2024 at 4:43 pm
submitted by /u/Hopeful-Candle-4884 [link] [comments]
Reddit Science This community is a place to share and discuss new scientific research. Read about the latest advances in astronomy, biology, medicine, physics, social science, and more. Find and submit new publications and popular science coverage of current research.
- Human decision makers who possess the authority to override ML predictions may impede the self-correction of discriminatory models and even induce initially unbiased models to become discriminatory with timeby /u/f1u82ypd on July 26, 2024 at 6:29 pm
submitted by /u/f1u82ypd [link] [comments]
- Study uses Game of Thrones (GOT) to advance understanding of face blindness: Psychologists have used the TV series GOT to understand how the brain enables us to recognise faces. Their findings provide new insights into prosopagnosia or face blindness, a condition that impairs facial recognition.by /u/AnnaMouse247 on July 26, 2024 at 5:14 pm
submitted by /u/AnnaMouse247 [link] [comments]
- Specific genes may be related to the trajectory of recovery for stroke survivors, study finds. Researchers say genetic variants were strongly associated with depression, PTSD and cognitive health outcomes. Findings may provide useful insights for developing targeted therapies.by /u/AnnaMouse247 on July 26, 2024 at 5:08 pm
submitted by /u/AnnaMouse247 [link] [comments]
- New experimental drug shows promise in clearing HIV from brain: originally developed to treat cancer, study finds that by targeting infected cells in the brain, drug may clear virus from hidden areas that have been a major challenge in HIV treatment.by /u/AnnaMouse247 on July 26, 2024 at 4:57 pm
submitted by /u/AnnaMouse247 [link] [comments]
- Rapid diagnosis sepsis tests could decrease result wait times from days to hours, researchers report in Natureby /u/Science_News on July 26, 2024 at 3:50 pm
submitted by /u/Science_News [link] [comments]
Reddit Sports Sports News and Highlights from the NFL, NBA, NHL, MLB, MLS, and leagues around the world.
- Charles Barkley leaves door open to post-TNT job optionsby /u/PrincessBananas85 on July 26, 2024 at 8:47 pm
submitted by /u/PrincessBananas85 [link] [comments]
- Report: Nuggets sign Westbrook to 2-year, $6.8M dealby /u/Oldtimer_2 on July 26, 2024 at 8:13 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Dolphins signing Tua to 4-year, $212.4M extensionby /u/Oldtimer_2 on July 26, 2024 at 8:09 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Rams cornerback Derion Kendrick suffers season-ending torn ACLby /u/Oldtimer_2 on July 26, 2024 at 8:06 pm
submitted by /u/Oldtimer_2 [link] [comments]
- Hosting the Olympics has become financially untenable, economists sayby /u/toaster_strudel_ on July 26, 2024 at 7:34 pm
submitted by /u/toaster_strudel_ [link] [comments]